


Reader.ReadString May Preserve Leading Delimiters
In Go, bufio.Reader.ReadString behavior can differ when parsing input. Consider the following scenario:
import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) fmt.Print("Who are you? Enter your name: ") text, _ := reader.ReadString('\n') if text == "Alice" || text == "Bob" { fmt.Printf("Hello, ", text) } else { fmt.Printf("You're not allowed in here!") } }
Here, ReadString is used to read user input and expects names terminated by a newline character. However, when the entered name matches "Alice" or "Bob," the program erroneously denies access.
Cause and Solution
This issue arises because ReadString preserves leading occurrences of the delimiter (in this case, 'n'). When a name like "Alice" is entered, the string stored in text includes both "Alice" and a trailing newline. This extra newline character prevents the string comparison from matching the expected value.
To resolve this issue, consider the following approaches:
- Trim Leading Whitespace: Before comparing text with "Alice" or "Bob," use strings.TrimSpace(text) to remove any leading whitespace, including the newline character.
- Use ReadLine Instead: Alternatively, switch to reader.ReadLine() instead of ReadString. This function returns the input without including any leading or trailing delimiters. Note that reader.ReadLine() will return bytes; hence, cast it to a string using string(bytes).
The above is the detailed content of Why Does Go's `bufio.Reader.ReadString` Preserve Leading Delimiters, and How Can I Avoid This Issue?. For more information, please follow other related articles on the PHP Chinese website!
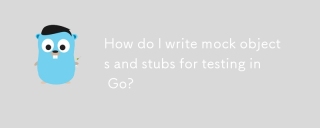
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
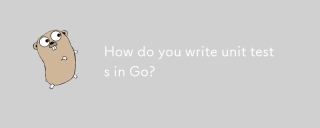
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
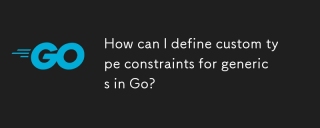
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
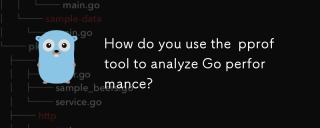
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
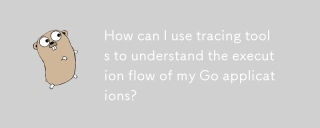
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
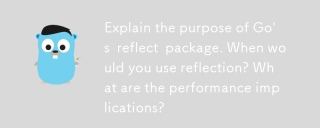
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
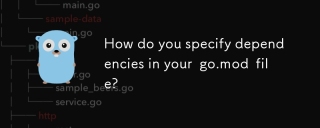
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
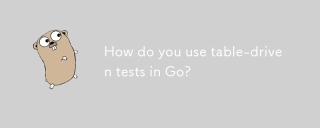
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
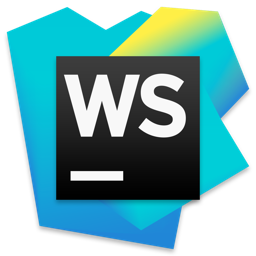
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
