


Range References Instead Values
In Go, the range keyword iterates over a sequence, such as an array or a slice, and provides access to both the key (index) and the value of each element. However, for performance reasons, the range keyword operates on a copy of the original value. This means that any modifications made to the value returned by range will not be reflected in the original array.
Example
Consider the following code:
package main import "fmt" type MyType struct { field string } func main() { var array [10]MyType // Attempting to modify a value returned by range for _, e := range array { e.field = "foo" } // Printing the values after the range loop for _, e := range array { fmt.Println(e.field) fmt.Println("--") } }
Running this code will print "000000" for all elements because the modifications made to e.field inside the first range loop are applied to a copy and do not affect the original value in the array.
Solution
To modify the values of an array using a range loop, you need to use the array index instead of the value returned by range. This can be achieved by capturing the index using the _ placeholder in the range loop.
package main import "fmt" type MyType struct { field string } func main() { var array [10]MyType // Using the array index to modify values for idx, _ := range array { array[idx].field = "foo" } // Printing the values after the range loop for _, e := range array { fmt.Println(e.field) fmt.Println("--") } }
This modified code will successfully change the values of the field for all elements in the array and print "foo" for each element.
The above is the detailed content of Why Doesn't Modifying Values in Go's Range Loop Change the Original Array?. For more information, please follow other related articles on the PHP Chinese website!
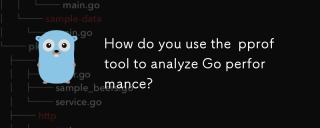
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
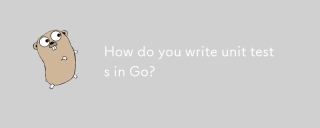
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
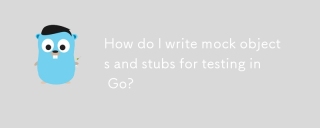
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
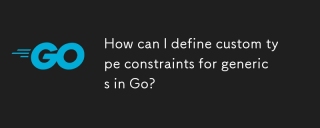
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
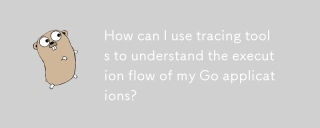
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
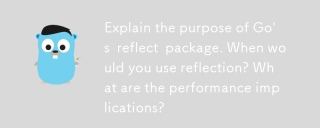
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
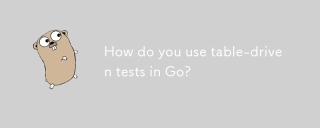
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
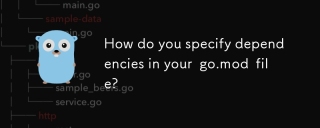
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
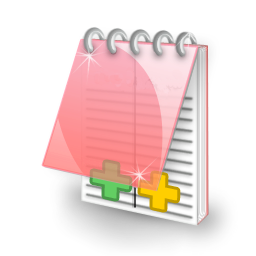
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
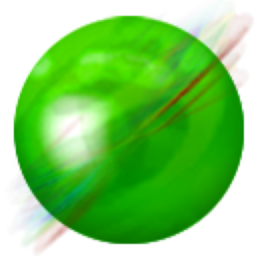
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
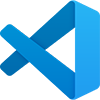
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
