


Executing Dynamic Queries and Retrieving Output in MySQL Stored Procedures
In MySQL, stored procedures allow you to perform complex operations efficiently. One common scenario is to dynamically generate queries based on input parameters and retrieve the result into a variable.
To achieve this, you can utilize prepared statements and output parameters. Let's consider the following stored procedure:
CREATE PROCEDURE 'searchInvoice' ( OUT numOfRecords INT ) BEGIN DECLARE query1 TEXT; DECLARE query2 TEXT; SET query1 = 'SELECT COUNT(*) bla bla bla.....'; // Query1 to select the count of matching tuples.. SET query2 = 'SELECT * from bla bla bla....'; // Query2 to select original records... // later part of this both queries generate dynamically according to some IN parameters.. // now I wanna assign the output of the query1 into numOfRecords // and I wanna execute the query2 as well.. like this SET @Sql = query2; PREPARE STMT FROM @Sql; EXECUTE STMT; DEALLOCATE PREPARE STMT; // output of the query2 can be read in PHP END
To retrieve the output of query1 into the numOfRecords parameter, you can use the following approach:
CREATE TABLE table1( column1 VARCHAR(255) DEFAULT NULL, column2 VARCHAR(255) DEFAULT NULL, column3 VARCHAR(255) DEFAULT NULL ); INSERT INTO table1 VALUES ('1', 'value1', 'value2'), ('2', 'value3', 'value4'); DELIMITER $$ CREATE PROCEDURE procedure1(IN Param1 VARCHAR(255), OUT Param2 VARCHAR(255), OUT Param3 VARCHAR(255)) BEGIN SET @c2 = ''; SET @c3 = ''; SET @query = 'SELECT column2, column3 INTO @c2, @c3 FROM table1 WHERE column1 = ?'; PREPARE stmt FROM @query; SET @c1 = Param1; EXECUTE stmt USING @c1; DEALLOCATE PREPARE stmt; SET Param2 = @c2; SET Param3 = @c3; END$$ DELIMITER ; -- Call procedure and use variables SET @Param1 = 2; SET @Param2 = ''; SET @Param3 = ''; CALL procedure1(@Param1, @Param2, @Param3); SELECT @Param2, @Param3;
In this example:
- The SET @c2 = '' and SET @c3 = '' statements initialize the output parameters.
- The SET @query = 'SELECT ...' statement prepares the query dynamically.
- The SET @c1 = Param1 statement assigns the input parameter to the query.
- The EXECUTE stmt USING @c1 statement executes the prepared query.
- The DEALLOCATE PREPARE stmt statement releases the prepared statement resources.
- The SET Param2 = @c2 and SET Param3 = @c3 statements assign the output values to the parameters.
- Finally, the SELECT @Param2, @Param3 statement retrieves the output values.
By utilizing this technique, you can retrieve the output of a dynamically generated query into a variable within a MySQL stored procedure.
The above is the detailed content of How to Retrieve Output from Dynamic Queries within MySQL Stored Procedures?. For more information, please follow other related articles on the PHP Chinese website!
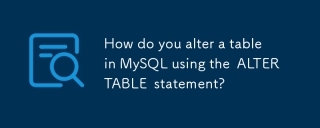
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
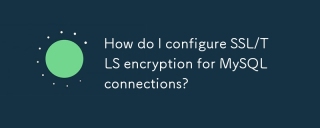
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
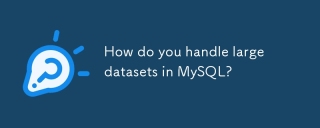
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
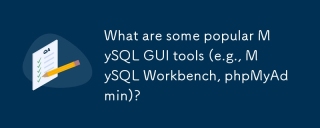
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
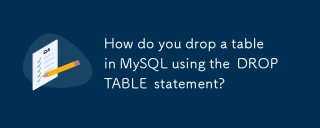
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
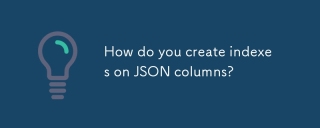
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
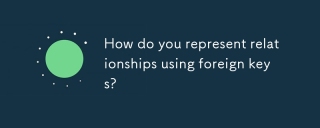
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
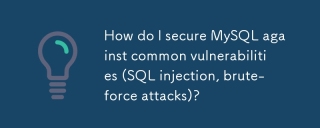
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
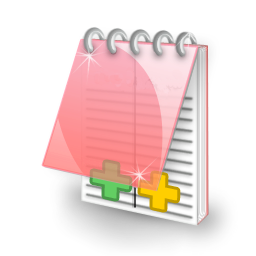
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
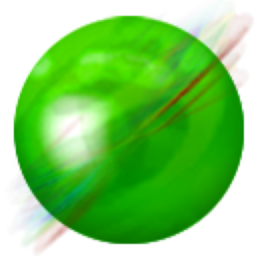
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
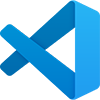
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
