


How Does JavaScript's Debounce Function Optimize Performance by Delaying Function Execution?
JavaScript's Debounce Function: A Comprehensive Guide
Understanding the "debounce" function in JavaScript can be puzzling. In essence, it delays the execution of a function until a specified amount of time has passed since the last call. This technique is commonly used to optimize performance and avoid unnecessary tasks.
How it Works
The debounce function in the provided code snippet operates as follows:
- Private Timer: It maintains a timeout variable that serves as an internal timer.
- Return Function: Debounce returns a new anonymous function that captures the context and arguments of the original function but introduces a delay.
- Immediate Mode: If immediate mode is enabled (immediate is true), the function is executed immediately if no timeout is active.
- Delay Timer: If the timeout is active, it is cleared. This cancels any pending execution and resets the timer. A new timeout is then set with the specified delay (wait).
- Execution: During the delay period, if the function is called again, the timer resets. Once the delay expires, the function is executed with the args and context captured at the time of invocation.
- Immediate Mode Execution: If immediate mode is true and no timeout is active, the function is executed immediately after the return function is called.
Example
Consider the example code snippet where mouse movements are debounced with a 50ms delay. When the mouse is moved, the console is cleared and the cursor's X and Y coordinates are logged. Without debouncing, this would result in numerous unnecessary calls to the console.log function, potentially slowing down performance.
function onMouseMove(e){ console.clear(); console.log(e.x, e.y); } // Define the debounced function var debouncedMouseMove = debounce(onMouseMove, 50); // Call the debounced function on every mouse move window.addEventListener('mousemove', debouncedMouseMove);
In this example, the debounced function optimizes the console activity by executing the clear and log operations only after a 50ms delay since the last mouse movement.
The above is the detailed content of How Does JavaScript's Debounce Function Optimize Performance by Delaying Function Execution?. For more information, please follow other related articles on the PHP Chinese website!
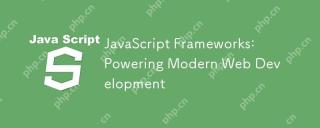
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
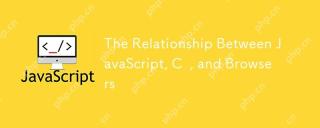
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
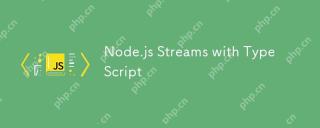
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
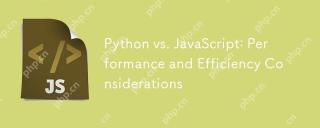
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
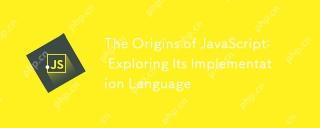
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
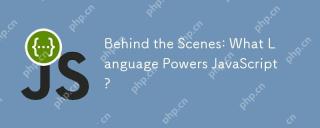
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
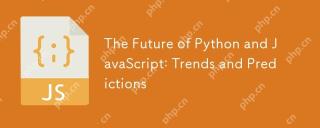
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
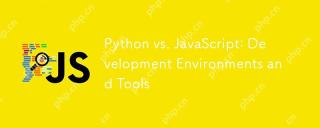
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
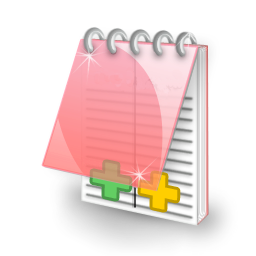
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
