


Loading Cross-Domain Endpoint with AJAX
Cross-Origin Resource Sharing (CORS) is a protocol that allows web browsers to make requests to resources on other domains. However, there are some restrictions in place to prevent malicious use of this capability.
Issue
When attempting to load a cross-domain HTML page using AJAX, you may encounter issues unless the dataType is set to "jsonp". However, using JSONP, the browser expects a script mime type, but it receives "text/html" instead. Additionally, using the crossDomain parameter has not resolved the issue.
Solution
There are several approaches to overcoming the cross-domain barrier:
JSONP
JSONP (JSON with Padding) is a technique that allows for cross-domain AJAX requests by wrapping the response in a function call. This can be achieved by setting the dataType parameter to "jsonp" and providing a callback function as the success handler.
$.ajax({ type: "GET", url: "crossdomainendpoint.com", dataType: "jsonp", success: function(data) { // Handle the JSONP response } });
CORS Proxy
CORS proxies are intermediary servers that can be used to bypass the same-origin policy. They add the necessary headers to the request to allow the browser to access resources on other domains. Several reputable CORS proxy services are available online.
$.ajax({ type: "GET", url: "https://cors-proxy.com/crossdomainendpoint.com", dataType: "json", success: function(data) { // Handle the CORS response } });
CORS Anywhere
CORS Anywhere is a popular CORS proxy server that can be used to fetch resources from any domain.
$.ajaxPrefilter(function(options) { if (options.crossDomain && $.support.cors) { options.url = "https://cors-anywhere.herokuapp.com/" + options.url; } }); $.ajax({ type: "GET", url: "crossdomainendpoint.com", dataType: "json", success: function(data) { // Handle the CORS response } });
Whatever Origin
Whatever Origin is an open-source library that uses JSONP to enable cross-domain requests.
$.ajax({ type: "GET", url: "http://whateverorigin.org/get?url=" + encodeURIComponent("crossdomainendpoint.com"), dataType: "jsonp", success: function(data) { // Handle the JSONP response } });
Note that while these techniques can help overcome cross-domain restrictions, it's important to consider security implications and adhere to the principles of Same-Origin Policy when working with external resources.
The above is the detailed content of How Can I Solve Cross-Domain AJAX Requests When Loading an HTML Endpoint?. For more information, please follow other related articles on the PHP Chinese website!
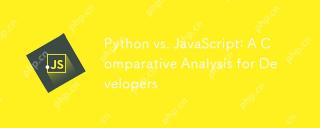
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
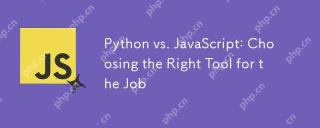
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
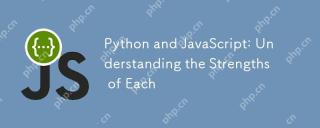
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
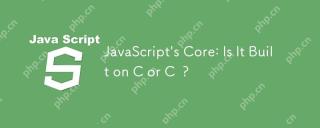
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
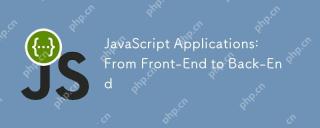
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
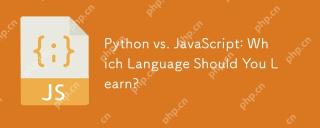
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
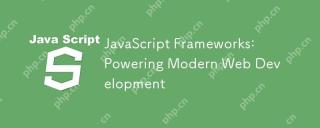
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
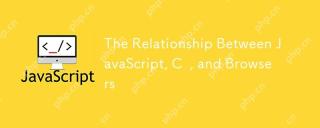
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
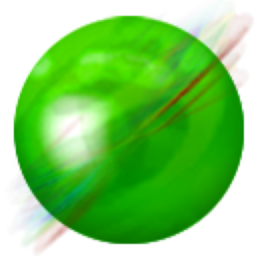
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
