Artwork: https://code-art.pictures/
Imagine a library that:
- Like Ramda, provides many useful functions for iterables manipulation
- Reads familiar, like standard Array iterative methods
- Doesn't try to replace JavaScript built-ins like Array, Set, or the iteration protocol
- Like Java Streams, includes Optional to clearly distinguish between “no value” and “undefined as a value”
- Is compact in terms of bundle size
I couldn't find one that fits all these needs, so I created my small library — fluent-streams.
Talk is Cheap. Show Me the Code
Top 3 Words in a Text
const words = ['Lorem', 'ipsum', /* ... */] stream(words) .groupBy(word => word.toLowerCase()) .map(([word, list]) => [word, list.length]) .sortBy(([, length]) => -length) .take(3) .toArray() // => ['ut', 3], ['in', 3], ['dolor', 2]
Coprime Integers
// Endless stream of 2..999 integers const randomInts = continually(() => 2 + Math.floor(Math.random() * 998) ) randomInts .zip(randomInts) .filter(([a, b]) => gcd(a, b) === 1) .distinctBy(pair => stream(pair).sortBy(i => i).join()) .take(10) // => [804, 835], [589, 642], [96, 145], ...
- Streams can be endless, as shown, but you can limit them to the first n items using the take(n) method
- A stream can be reused multiple times, even in parallel. This is because streams are stateless and only store a reference to the input. The state is created only when the stream spawns an iterator.
Generate a Deck of Cards
const deck = streamOf('♠', '♥', '♣', '♦') .flatMap(suit => streamOf<string number>( 'A', ...range(2, 11), 'J', 'Q', 'K' ).map(rank => `${rank}${suit}`) ) // => 'A♠', '2♠', '3♠', ... </string>
And Play Hold'em Poker!
const playersNum = 2 const [flop, turn, river, ...hands] = deck .takeRandom(3 + 1 + 1 + playersNum * 2) .zipWithIndex() .splitWhen((_, [, j]) => j === 3 // flop || j === 4 // turn || j >= 5 // river && j % 2 === 1 // ...players' hands ) .map(chunk => // Unzip index chunk.map(([card]) => card) ) // flop = ['3♦', '9♣', 'J♦'] // turn = ['4♣'] // river = ['7♦'] // hands = ['J♠', '4♥'], ['10♠', '8♥']
First player has a pair of Jacks on the flop, while the second player gets a straight, but only on the river. Who will win?
This Must Be Cheap
Everything above can be achieved with only native data structures. However, code written with Fluent Streams reads nicer. While making code more readable is a perfectly valid goal, the cost of achieving it should be low in terms of cognitive load, bundle size, and performance.
And that’s exactly the case with Fluent Streams! Here’s why:
- No learning curve: The API feels familiar, resembling standard Array methods. It’s easy to add and just as easy to remove.
- No reinvention: The library doesn’t create new data structures or protocols — it builds on JavaScript’s already robust features.
- Minimal bundle impact: At just 8.5 kB minified, it’s lightweight. If your project already includes React and its satellite libraries, which weigh hundreds of kilobytes, this addition is hardly noticeable.
- Lazy processing: The library processes items lazily, which can reduce memory usage and improve efficiency in long pipelines by avoiding unnecessary intermediate data copying.
Caveats
The library is shipped untranspiled to ES5. This decision is driven by the desire to maintain a small bundle size, which is achieved by leveraging ES6 features that enable iteration with very concise code — most notably generators. However, only widely supported language features are utilized.
If you are still transpiling to ES5, you can use the library by transpiling it yourself and adding polyfills. Be aware, though, that this will increase the bundle size, so it’s not recommended. Instead, this might be the perfect occasion to revisit your build configuration and embrace modern JavaScript features.
Have Fun Coding!
The above is the detailed content of Fluent Streams: A Library for Rich Iterables Manipulation. For more information, please follow other related articles on the PHP Chinese website!
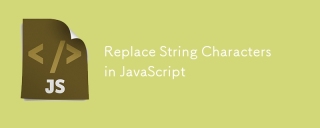
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
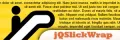
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
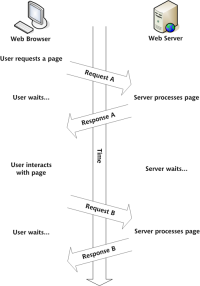
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
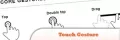
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
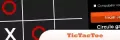
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
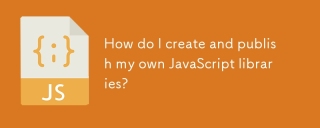
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
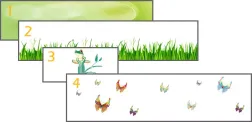
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
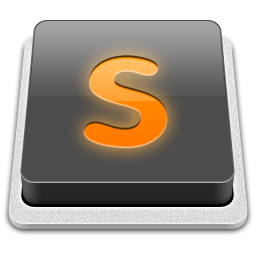
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
