How Can I Effectively Validate Dates in Java Without Using Deprecated Methods?
Validating Dates in Java: Debunking the Deprecated Approach
Java's once-common practice of creating Date objects using the Date class has become outdated. This has left developers pondering the best method for verifying the validity of dates expressed as combinations of day, month, and year. Take the example of "2008-02-31," which is a seemingly invalid date.
A Simple and Effective Solution
To address this challenge, a remarkably straightforward solution exists. By utilizing the SimpleDateFormat class in conjunction with the setLenient method set to false, you gain the ability to meticulously scrutinize dates for their legitimacy.
Implementation
The following Java code snippet demonstrates the application of this approach:
import java.text.ParseException; import java.text.SimpleDateFormat; public class DateValidation { public static void main(String[] args) { String date = "2008-02-31"; SimpleDateFormat df = new SimpleDateFormat("dd-MM-yyyy"); df.setLenient(false); try { df.parse(date); System.out.println("Valid date"); } catch (ParseException e) { System.out.println("Invalid date"); } } }
By setting the lenient flag to false, the parse method will strictly adhere to the predefined date format. Any deviation from a valid date, such as "2008-02-31," will result in a ParseException exception.
While this approach provides a straightforward means of date validation, it may not be suitable for all scenarios. For more comprehensive date handling and validation needs, consider exploring alternative libraries like Joda-Time or the newer Time API introduced in Java 8.
The above is the detailed content of How Can I Effectively Validate Dates in Java Without Using Deprecated Methods?. For more information, please follow other related articles on the PHP Chinese website!
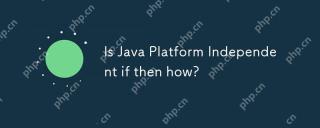
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
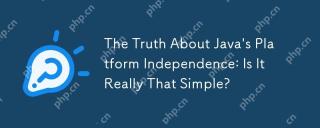
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
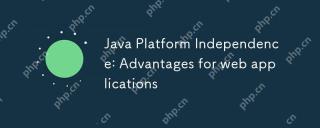
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
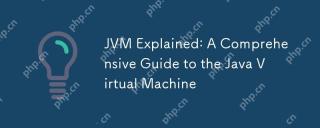
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
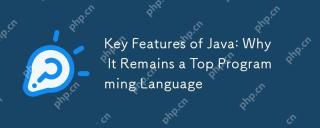
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
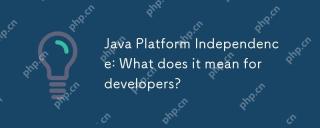
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
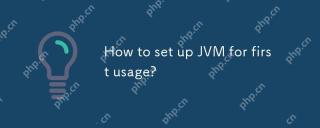
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
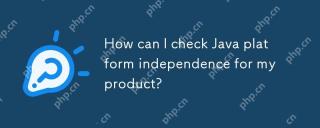
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
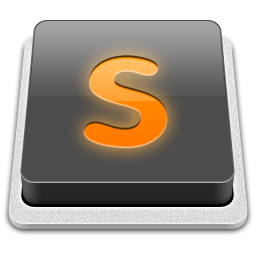
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment
