Understanding the Difference Between reflect.ValueOf() and Value.Elem() in Go
When exploring Go's reflection capabilities, distinguishing between reflect.ValueOf() and Value.Elem() is crucial. Both return Value objects, but their specific purposes and use cases differ.
reflect.ValueOf()
reflect.ValueOf() is a function that takes an interface{} value and returns a Value object representing that value. It is used to create a reflection descriptor for a non-reflection value, such as an integer or a string.
Value.Elem()
Value.Elem() is a method of the Value type, which represents a reflection object. It retrieves the value that the Value object points to or contains.
When to Use .Elem()
To determine when to use .Elem(), it's important to understand that a Value object can hold a pointer value or an interface value. Using .Elem() is appropriate in the following situations:
- Retrieving the pointed value: If you have a Value object that wraps a pointer to a value, v.Elem() will return the concrete value that the pointer refers to. This allows you to traverse the pointer chain to access the underlying value.
- Retrieving the underlying value in an interface: In Go, interfaces are essentially wrappers for concrete values. If you have a Value object that wraps an interface value, v.Elem() will return the underlying concrete value. This is useful for accessing the actual value stored within the interface.
Example Usage
Consider the following example, where we use .Elem() to retrieve the concrete value from an interface:
package main import ( "fmt" "reflect" ) type Person struct { Name string } func main() { // Create an interface value holding a Person value. pi := reflect.ValueOf(&Person{"Alice"}) // Use Elem() to retrieve the underlying Person value. p := pi.Elem() // Print the person's name. fmt.Println(p.FieldByName("Name").String()) }
Advanced Use Case for .Elem()
In a more advanced scenario, .Elem() can also be used to navigate a chain of pointers or interfaces. For instance, if you have a Value object representing a pointer to a pointer to an interface, you can use .Elem() multiple times to traverse the pointer chain and retrieve the underlying concrete value.
Conclusion
Understanding the subtle differences between reflect.ValueOf() and Value.Elem() is essential for effective use of reflection in Go. By applying these functions appropriately, you can manipulate and access values dynamically at runtime, providing flexibility and extensibility in your code.
The above is the detailed content of Go Reflection: When Should I Use `Value.Elem()`?. For more information, please follow other related articles on the PHP Chinese website!
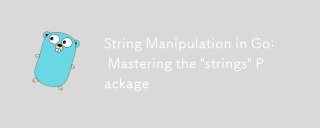
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
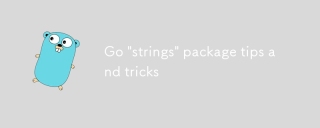
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
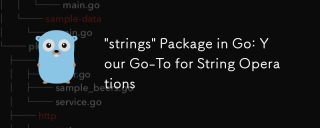
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
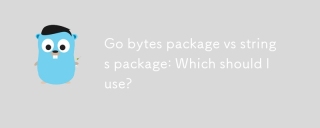
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
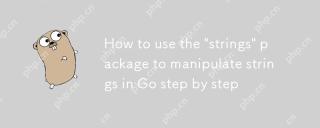
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
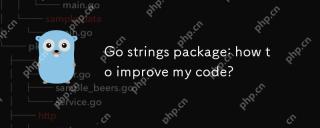
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
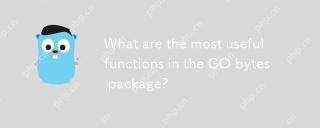
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
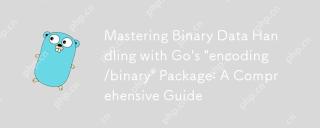
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
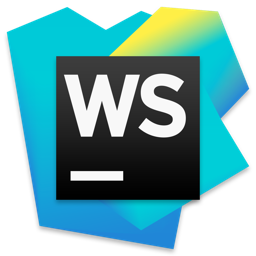
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
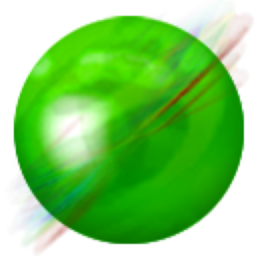
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
