


Use the remote Method in jQuery Validate to Verify Username Availability
Question:
Can you explain how to integrate jQuery Validate with a PHP script to check if a username already exists in a database?
Answer:
To perform this validation, follow these steps:
jQuery:
$("#signupForm").validate({ rules: { username: { required: true, minlength: 3, remote: "check-username.php" } }, messages: { username: { remote: "This username is already taken! Try another." } } });
check-username.php:
For this example, PHP is used for the database connectivity and validation:
<?php require_once "./source/includes/data.php"; header('Content-type: application/json'); $name = mysql_real_escape_string($_POST['username']); $query = mysql_query("SELECT * FROM mmh_user_info WHERE username ='$username'"); $result = mysql_num_rows($query); if ($result == 0){ $valid = 'true';} else{ $valid = 'false'; } echo $valid; ?>
Example Usage:
- This JavaScript will trigger the validation upon form submission:
$("#signupForm").submit(function(e) { e.preventDefault(); $(this).validate(); });
- The PHP script will check if the username exists in the database:
// Initialize the database connection // Query to check if the username exists $query = "SELECT * FROM mmh_user_info WHERE username='$name'"; $result = mysql_query($query); // Check the result and return a JSON response if (mysql_num_rows($result) > 0) { echo json_encode(array('status' => 'error', 'message' => 'Username already exists')); } else { echo json_encode(array('status' => 'success', 'message' => 'Username available')); }
The above is the detailed content of How to Use jQuery Validate's Remote Method to Check Username Availability?. For more information, please follow other related articles on the PHP Chinese website!
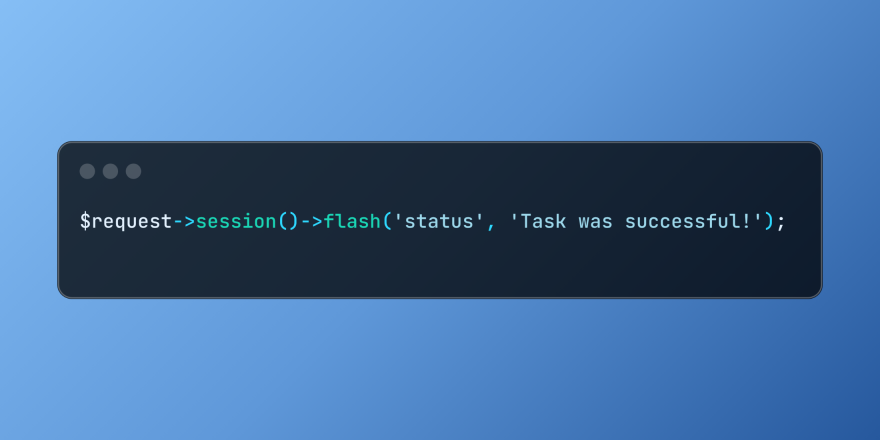
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
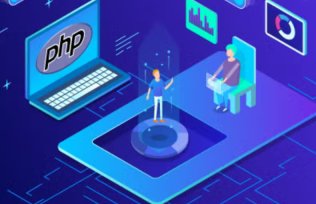
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
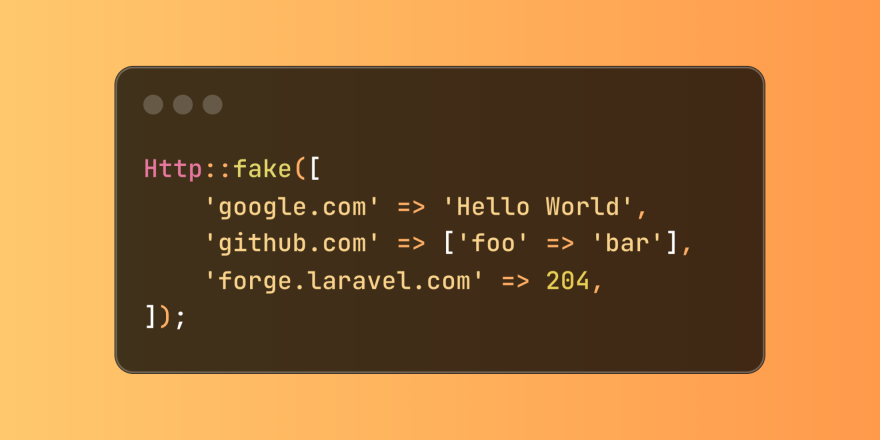
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
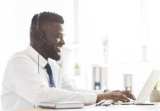
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
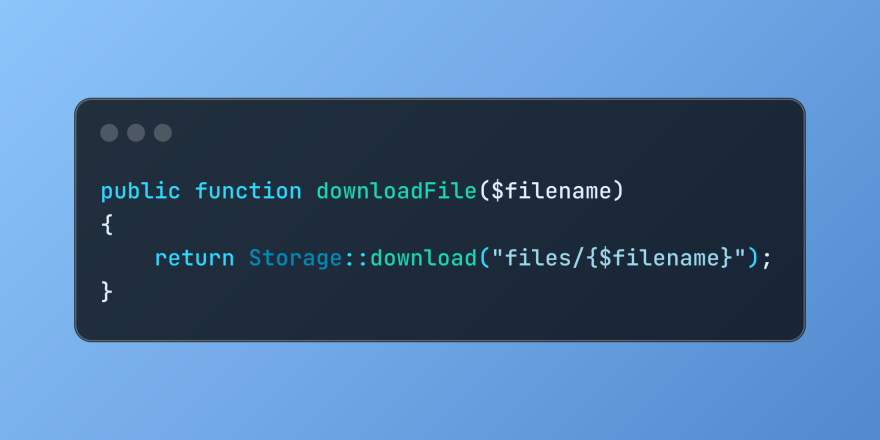
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
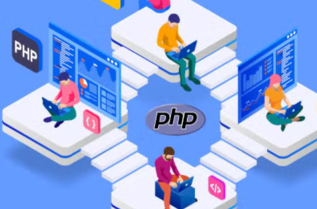
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
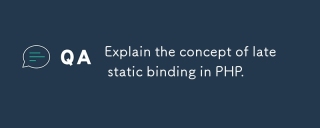
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
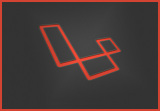
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
