


Generating All Combinations of Items in Multiple Arrays in PHP
The task of finding all combinations of items in multiple arrays is encountered often in programming. The number of arrays and elements within each array may vary.
Recursive Solution
A recursive approach can effectively address this problem. The combinations() function provides a solution:
function combinations($arrays, $i = 0) { if (!isset($arrays[$i])) { return array(); } if ($i == count($arrays) - 1) { return $arrays[$i]; } // get combinations from subsequent arrays $tmp = combinations($arrays, $i + 1); $result = array(); // concat each array from tmp with each element from $arrays[$i] foreach ($arrays[$i] as $v) { foreach ($tmp as $t) { $result[] = is_array($t) ? array_merge(array($v), $t) : array($v, $t); } } return $result; }
Example Usage
Consider the arrays:
$arrayA = array('A1','A2','A3'); $arrayB = array('B1','B2','B3'); $arrayC = array('C1','C2');
To find all combinations:
print_r(combinations(array($arrayA, $arrayB, $arrayC)));
Output:
Array ( [0] => A1 [1] => B1 [2] => C1 [3] => A1 [4] => B1 [5] => C2 [6] => A1 [7] => B2 [8] => C1 [9] => A1 [10] => B2 [11] => C2 [12] => A1 [13] => B3 [14] => C1 [15] => A1 [16] => B3 [17] => C2 [18] => A2 [19] => B1 [20] => C1 [21] => A2 [22] => B1 [23] => C2 [24] => A2 [25] => B2 [26] => C1 [27] => A2 [28] => B2 [29] => C2 [30] => A2 [31] => B3 [32] => C1 [33] => A2 [34] => B3 [35] => C2 [36] => A3 [37] => B1 [38] => C1 [39] => A3 [40] => B1 [41] => C2 [42] => A3 [43] => B2 [44] => C1 [45] => A3 [46] => B2 [47] => C2 [48] => A3 [49] => B3 [50] => C1 [51] => A3 [52] => B3 [53] => C2 )
This solution provides all possible combinations in an efficient manner.
The above is the detailed content of How Can I Generate All Combinations of Items from Multiple Arrays in PHP?. For more information, please follow other related articles on the PHP Chinese website!
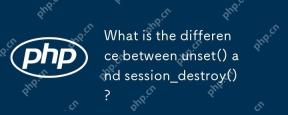
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
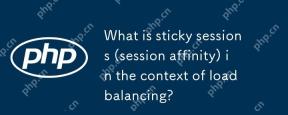
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
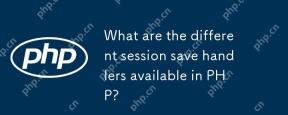
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
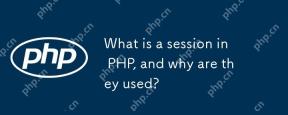
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
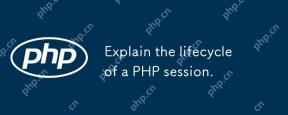
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
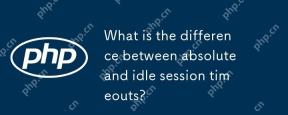
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
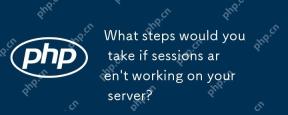
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
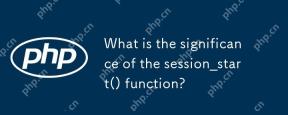
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
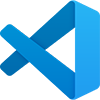
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
