Build a Simple Chatbot with Svelte and ElizaBot
Have you ever wanted to create a simple chatbot? In this article, we'll use Svelte and the classic ElizaBot to build a lightweight chatbot application. You'll learn how to handle user interactions, manage reactive state, and even simulate a typing delay for a realistic experience.
Let's dive in!
Prerequisites
To follow along, you'll need:
- Basic knowledge of Svelte.
- A working environment to build Svelte apps. If you don't have one, check out the Svelte Getting Started guide.
Setting Up the Project
Start by installing ElizaBot, a simple chatbot library:
npm install elizabot
Create a new Svelte app using your preferred method, and then include the following code in a Svelte component file (e.g., Chatbot.svelte).
The Code
Here’s the complete code for the chatbot:
Script
<script> import Eliza from 'elizabot'; import { afterUpdate, beforeUpdate } from 'svelte'; let div; let autoscroll; const eliza = new Eliza(); let comments = [{ author: 'eliza', text: eliza.getInitial() }]; function handleKeydown(event) { if (event.key === 'Enter') { const text = event.target.value.trim(); if (!text) return; comments = comments.concat({ author: 'user', text }); event.target.value = ''; const reply = eliza.transform(text); setTimeout(() => { comments = comments.concat({ author: 'eliza', text: '...', placeholder: true }); setTimeout(() => { comments = comments.filter((comment) => !comment.placeholder).concat({ author: 'eliza', text: reply }); }, Math.random() * 500); }, Math.random() * 200); } } beforeUpdate(() => { autoscroll = div && (div.offsetHeight + div.scrollTop) > (div.scrollHeight - 20); }); afterUpdate(() => { if (autoscroll) div.scrollTo(0, div.scrollHeight); }); </script>
Styling
<style> .chat { display: flex; flex-direction: column; height: 100%; max-width: 320px; } .scrollable { flex: 1 1 auto; border-top: 1px solid #eee; margin: 0 0 0.5em 0; overflow-y: auto; } article { margin: 0.5em 0; } .user { text-align: right; } span { padding: 0.5em 1em; display: inline-block; } .eliza span { background-color: #eee; border-radius: 1em 1em 1em 0; } .user span { background-color: #ea0a0a; color: white; border-radius: 1em 1em 0 1em; word-break: break-all; } </style>
HTML Markup
<div> <hr> <h2> How It Works </h2> <ol> <li> <p><strong>User Input Handling</strong>:</p> <ul> <li>When the user types a message and presses "Enter," it's added to the comments array with the author set as user.</li> </ul> </li> <li> <p><strong>ElizaBot Reply</strong>:</p> <ul> <li>The message is passed to ElizaBot for a response using eliza.transform(text).</li> <li>A placeholder message (...) is shown during the simulated typing delay. The final response is then displayed after a short random delay to simulate typing.</li> </ul> </li> <li> <p><strong>Auto-Scroll</strong>:</p> <ul> <li>The beforeUpdate and afterUpdate lifecycle hooks ensure the chat automatically scrolls to the latest message unless the user manually scrolls up.</li> </ul> </li> <li> <p><strong>Styling</strong>:</p> <ul> <li>Messages from the bot and user are styled differently using dynamic CSS classes (eliza and user). This helps visually distinguish between the bot's responses and the user's input.</li> </ul> </li> </ol> <hr> <h2> Running the App </h2> <p>Start your Svelte app, and you'll see a chat interface with ElizaBot. Try typing messages and watch the bot respond. The delay gives a natural feel to the interaction.</p> <hr> <h2> Enhancements </h2> <p>Want to take this further? Here are some ideas:</p> <ul> <li> <strong>Add a Send Button</strong>: Include a button to send messages in addition to pressing "Enter." This can improve accessibility and user experience.</li> <li> <strong>Keyword-Specific Responses</strong>: Enhance ElizaBot's responses by adding custom logic for certain keywords or phrases.</li> <li> <strong>Message Timestamps</strong>: Display timestamps for each message to give the chat a more realistic feel.</li> <li> <strong>Persist Chat History</strong>: Save the chat history in local storage or a database so users can revisit their previous conversations.</li> <li> <strong>Typing Animation</strong>: Add an animation or spinner for ElizaBot to make the typing delay more visually engaging.</li> <li> <strong>Mobile Responsiveness</strong>: Ensure the chat interface adapts well to different screen sizes for a seamless experience on mobile devices.</li> </ul> <hr> <h2> Conclusion </h2> <p>In this tutorial, we built a simple chatbot using Svelte and ElizaBot. This example demonstrates Svelte’s reactivity and lifecycle hooks in action, while also providing a fun and interactive way to experiment with chatbots.</p> </div>
The above is the detailed content of Build a Simple Chatbot with Svelte and ElizaBot. For more information, please follow other related articles on the PHP Chinese website!
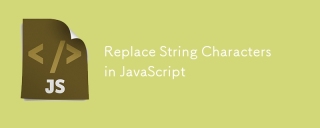
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
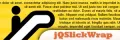
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
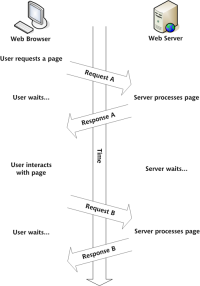
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
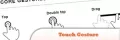
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
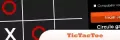
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
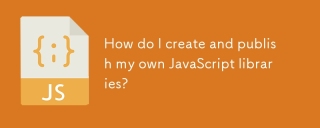
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
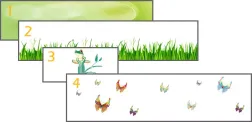
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
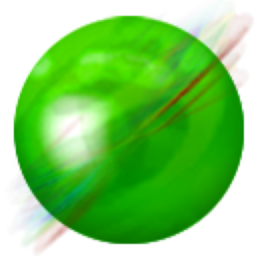
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
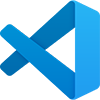
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
