How to define and use a foreign key constraint in GORM?
In the realm of database design, foreign keys play a crucial role in establishing relationships and maintaining data integrity. For GORM users, understanding how to define and use foreign key constraints is essential.
Understanding the Problem
Consider the scenario described in the question, where we have two models: User and UserInfo. We need to establish a foreign key relationship between the UID field in UserInfo and the id field in User.
GORM's Foreign Key Generation
In GORM versions prior to 2.0, we needed to manually define foreign key constraints using the AddForeignKey method. In GORM 2.0 and later, this step is no longer necessary.
// GORM version 1.x db.Model(&models.UserInfo{}).AddForeignKey("u_id", "t_user(id)", "RESTRICT", "RESTRICT")
Automated Foreign Key Definition
However, for older versions of GORM, we can still define foreign keys as shown below.
type User struct { DBBase Email string `gorm:"column:email" json:"email"` Password string `gorm:"column:password" json:"-"` } func (User) TableName() string { return "t_user" } type UserInfo struct { User User `gorm:"foreignkey:u_id;association_foreignkey:id"` UID uint `gorm:"column:u_id" json:"-"` FirstName string `gorm:"column:first_name" json:"first_name"` LastName string `gorm:"column:last_name" json:"last_name"` Phone string `gorm:"column:phone" json:"phone"` Address string `gorm:"column:address" json:"address"` } func (UserInfo) TableName() string { return "t_user_info" }
Note that in this example, we define the foreign key relationship menggunakan the gorm:"foreignkey:..." and gorm:"association_foreignkey:..." annotations.
Setting Foreign Key Values in Code
Next, let's address the issue of setting foreign key values correctly. In the provided code:
func (dao *AuthDAO) Register(rs app.RequestScope, user *models.User, userInfo *models.UserInfo) (userErr error, userInfoErr error) { createUser := rs.Db().Create(&user) userInfo.UID = user.ID createUserInfo := rs.Db().Create(&userInfo) return createUser.Error, createUserInfo.Error }
We set userInfo.UID to user.ID after creating the User instance. This approach is valid and will correctly establish the foreign key relationship.
In conclusion, understanding foreign key relationships and their implementation in GORM is crucial for maintaining data integrity in database-driven applications. By following the suggested solutions and annotations, you can effectively define and utilize foreign key constraints in your GORM models.
The above is the detailed content of How to Define and Use Foreign Key Constraints in GORM?. For more information, please follow other related articles on the PHP Chinese website!
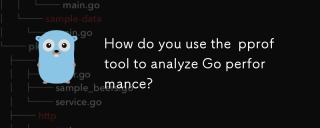
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
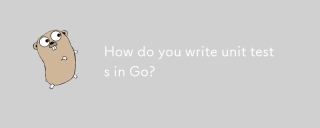
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
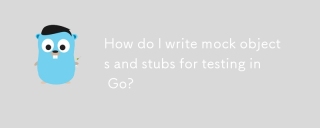
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
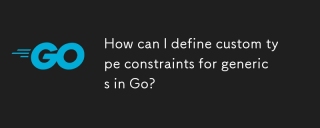
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
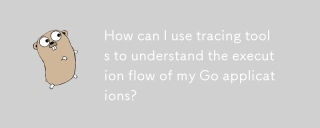
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
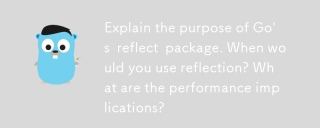
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
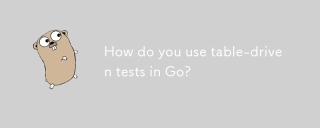
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
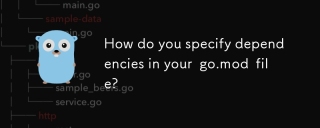
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
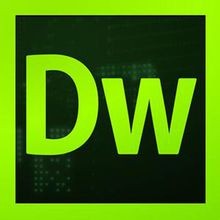
Dreamweaver CS6
Visual web development tools
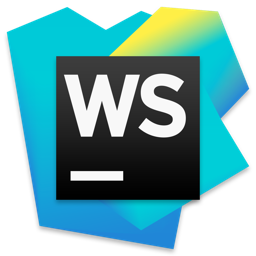
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor
