


Choosing Between bind_result and get_result
When working with prepared statements for database queries, selecting the appropriate method to handle the result can significantly impact the efficiency and flexibility of your code. This article examines the differences between bind_result and get_result, two methods commonly used to retrieve result data.
bind_result
bind_result is ideal when precise control over variable assignment is desired. By explicitly specifying the variables to be bound to each column, it ensures that the order of variables strictly matches the structure of the returned row. This approach is advantageous when the structure of the returned row is known in advance, and the code can be tailored accordingly.
$query1 = 'SELECT id, first_name, last_name, username FROM `table` WHERE id = ?'; $id = 5; $stmt = $mysqli->prepare($query1); /* Binds variables to prepared statement i corresponding variable has type integer d corresponding variable has type double s corresponding variable has type string b corresponding variable is a blob and will be sent in packets */ $stmt->bind_param('i',$id); /* execute query */ $stmt->execute(); /* Store the result (to get properties) */ $stmt->store_result(); /* Get the number of rows */ $num_of_rows = $stmt->num_rows; /* Bind the result to variables */ $stmt->bind_result($id, $first_name, $last_name, $username); while ($stmt->fetch()) { echo 'ID: '.$id.'<br>'; echo 'First Name: '.$first_name.'<br>'; echo 'Last Name: '.$last_name.'<br>'; echo 'Username: '.$username.'<br><br>'; }
Pros of bind_result:
- Compatible with outdated PHP versions
- Returns separate variables
Cons of bind_result:
- Manual listing of all variables required
- Requires more code to return the row as an array
- Code must be updated when the table structure changes
get_result
get_result provides a more versatile solution for data retrieval. It automatically creates an associative/enumerated array or object containing the data from the returned row. This method is convenient when dealing with dynamic result structures or when flexibility in accessing data is essential.
$query2 = 'SELECT * FROM `table` WHERE id = ?'; $id = 5; $stmt = $mysqli->prepare($query2); /* Binds variables to prepared statement i corresponding variable has type integer d corresponding variable has type double s corresponding variable has type string b corresponding variable is a blob and will be sent in packets */ $stmt->bind_param('i',$id); /* execute query */ $stmt->execute(); /* Get the result */ $result = $stmt->get_result(); /* Get the number of rows */ $num_of_rows = $result->num_rows; while ($row = $result->fetch_assoc()) { echo 'ID: '.$row['id'].'<br>'; echo 'First Name: '.$row['first_name'].'<br>'; echo 'Last Name: '.$row['last_name'].'<br>'; echo 'Username: '.$row['username'].'<br><br>'; }
Pros of get_result:
- Returns associative/enumerated array or object automatically filled with data
- Allows fetch_all() method to return all returned rows at once
Cons of get_result:
- Requires MySQL native driver (mysqlnd)
Conclusion
The choice between bind_result and get_result depends on the specific requirements of the application. bind_result provides precision and control over result data, but can be cumbersome for dynamic data structures. get_result offers flexibility and convenience, but may not be supported in older PHP versions. Understanding the advantages and limitations of each method allows developers to make informed decisions when handling query results.
The above is the detailed content of `bind_result` vs. `get_result`: Which MySQLi Method Should I Use for Retrieving Query Results?. For more information, please follow other related articles on the PHP Chinese website!
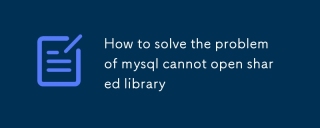
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
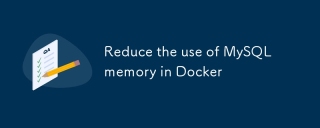
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
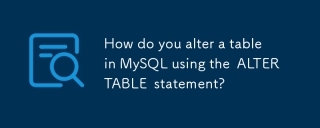
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
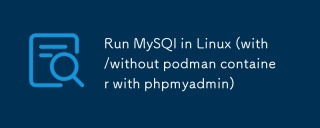
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
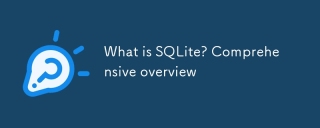
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
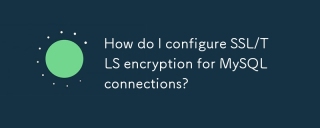
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
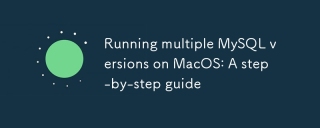
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
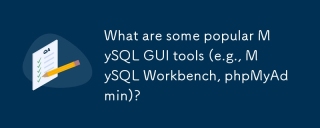
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
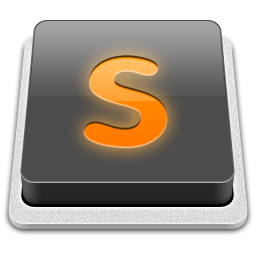
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
