How Can I Sort a List of Cars by a Custom Color Order Using Java Comparators?
Using Comparators for Custom Sorting
When implementing a sorting mechanism, it's crucial to define a custom sort order to suit specific requirements. In this context, Java provides Comparator to facilitate such operations.
In your example, you aim to sort a list of cars by their color, but not in alphabetical order. You desire a custom order where "Red" comes first, followed by "Blue" and so on.
To achieve this, the following steps can be implemented:
1. Define an Enum for Car Colors:
Create an enum named PaintColors to represent the available car colors. This approach ensures that the natural ordering of the enum corresponds to your desired sort order.
public enum PaintColors { SILVER, BLUE, MAGENTA, RED }
2. Modify the Car Class:
Instead of using strings for color, change Car to use the PaintColors enum.
public class CarSort { private String name; private PaintColors color; ... }
3. Implement a Comparator for Color Sorting:
Define a comparator class called ColorComparator to compare cars based on their colors.
static class ColorComparator implements Comparator<carsort> { public int compare(CarSort c1, CarSort c2) { return c1.getColor().compareTo(c2.getColor()); } }</carsort>
4. Customize Sorting in Main Method:
In the main method, modify the code to use Collections.sort with the ColorComparator:
Collections.sort(carList, new ColorComparator());
By implementing these steps, you can establish a custom sort order for your car list, allowing you to prioritize specific colors as needed.
The above is the detailed content of How Can I Sort a List of Cars by a Custom Color Order Using Java Comparators?. For more information, please follow other related articles on the PHP Chinese website!
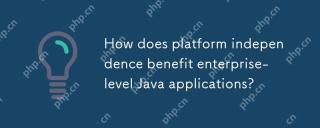
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
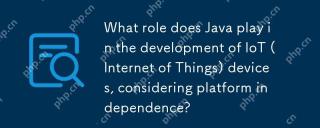
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
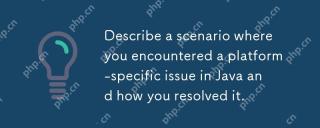
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
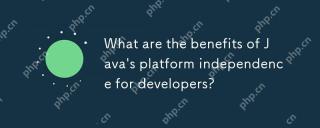
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
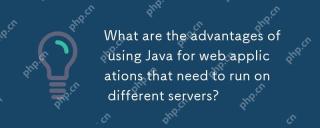
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
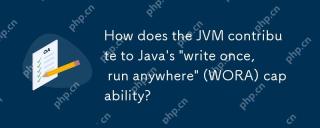
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
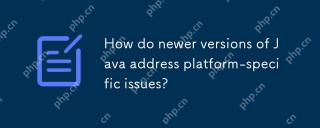
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
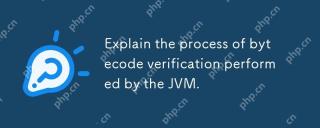
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
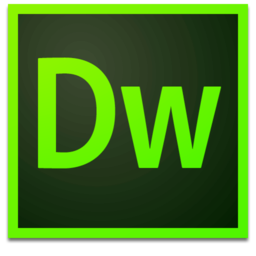
Dreamweaver Mac version
Visual web development tools
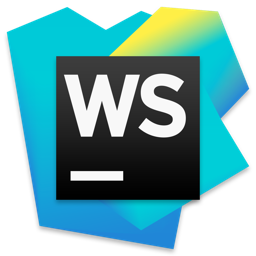
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
