


How to Sort Structures with Multiple Parameters
In programming, it's often necessary to sort data based on multiple criteria. In Go, this can be achieved effectively using custom sorting functions.
Problem:
How do you sort a slice of structs by LastName and then FirstName?
Solution using slices.SortFunc (Go 1.22 ):
slices.SortFunc(members, func(a, b Member) int { return cmp.Or( cmp.Compare(a.LastName, b.LastName), cmp.Compare(a.FirstName, b.FirstName), ) })
This solution uses the slices.SortFunc function to compare structs by their LastName and FirstName fields in that order.
Solution using sort.Slice or sort.Sort:
sort.Slice(members, func(i, j int) bool { if members[i].LastName != members[j].LastName { return members[i].LastName <pre class="brush:php;toolbar:false">type byLastFirst []Member func (members byLastFirst) Len() int { return len(members) } func (members byLastFirst) Swap(i, j int) { members[i], members[j] = members[j], members[i] } func (members byLastFirst) Less(i, j int) bool { if members[i].LastName != members[j].LastName { return members[i].LastName <p>Both solutions compare the LastName fields first. If they are equal, they compare the FirstName fields. The result is a sorted slice of members by both fields.</p>
The above is the detailed content of How to Sort Structs in Go by Multiple Fields (LastName then FirstName)?. For more information, please follow other related articles on the PHP Chinese website!
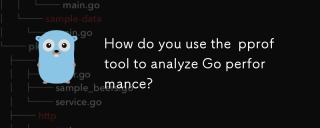
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
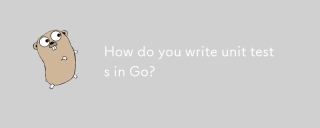
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
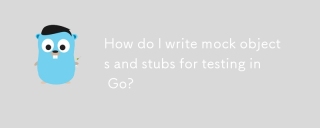
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
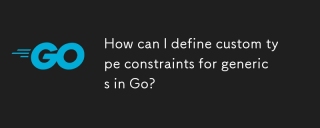
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
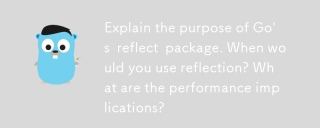
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
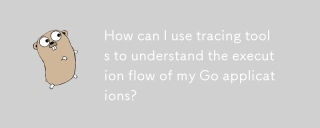
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
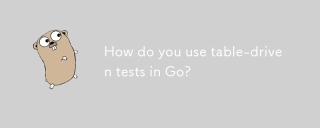
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
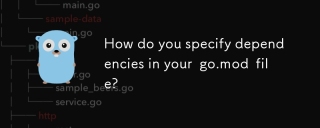
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
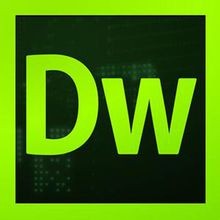
Dreamweaver CS6
Visual web development tools
