How to Address Alignment Issues with AVX Load/Store Operations
Problem:
When using YMM registers with AVX intrinsics, developers may encounter alignment issues, leading to a program crash when trying to store to a memory address that is not properly aligned to 32-byte boundaries. This alignment issue is caused by the fact that YMM registers require 32-byte alignment for optimal performance.
Workaround:
To resolve this issue, developers can utilize AVX unaligned load/store intrinsics _mm256_loadu_ps / storeu. These intrinsics allow data to be loaded or stored even if it is not properly aligned. While using unaligned memory access may lead to a slight performance penalty, it ensures that the program can run without crashing.
Best Practices:
For optimal performance, it is generally recommended to align data to 32-byte boundaries whenever possible. This can be achieved using alignas(32) when declaring arrays or structures. By default, new and malloc allocate memory with an alignment of max_align_t, which may be insufficient for AVX operations.
Alternatives:
- new(std::align_val_t(32)): In C 17 and above, this syntax can be used to explicitly allocate memory with 32-byte alignment.
- std::aligned_alloc(32, size): This function attempts to allocate memory with 32-byte alignment. However, it is important to note that it requires the size to be a multiple of 32.
- posix_memalign: This POSIX function can allocate memory with arbitrary alignment. However, it is not standardized and may not be available on all platforms.
- _mm_malloc: This Intel function allocates memory with 32-byte alignment. However, it is only compatible with Intel's MKL (_mm_whatever_ps) functions and not with standard C or C memory management functions.
- mmap / VirtualAlloc: System-level functions can be used to allocate memory with specific alignment and page permissions. This approach is typically recommended for large memory allocations.
Additional Considerations:
- Alignas on Arrays/Structs: In C 11 and later, alignas(32) can be used on arrays or struct members to enforce 32-byte alignment.
- Alignment in C 17: C 17 introduces automatic alignment for certain types like __m256, ensuring that they are allocated with the correct alignment.
- Trade-Off: It is important to balance alignment requirements with performance considerations. Unaligned memory access can lead to performance penalties, so it should only be used when necessary.
The above is the detailed content of How to Handle Alignment Issues When Using AVX Load/Store Operations?. For more information, please follow other related articles on the PHP Chinese website!
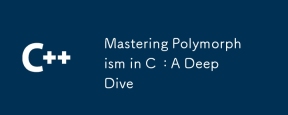
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
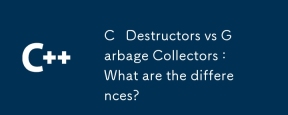
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
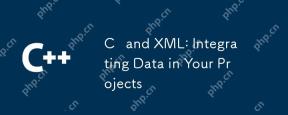
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
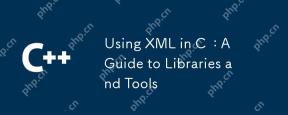
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
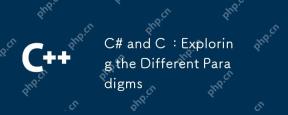
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
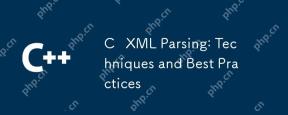
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
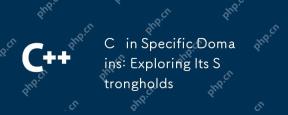
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
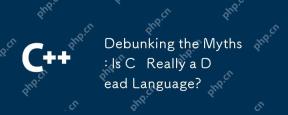
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
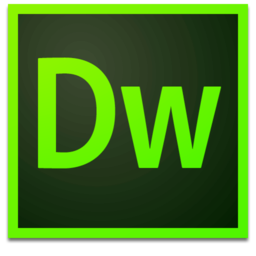
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
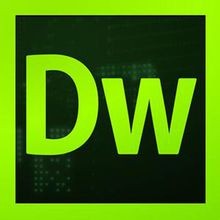
Dreamweaver CS6
Visual web development tools
