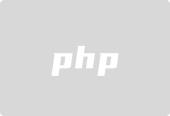
Creating an empty Pandas DataFrame, and then filling it
Iteratively filling a DataFrame with values
Using the given DataFrame documentation, you want to iteratively fill the DataFrame with values in a time series kind of calculation. The goal is to initialize the DataFrame with columns A, B, and timestamp rows, all 0 or all NaN. Then, you want to add initial values and go over this data calculating the new row from the row before, say row[A][t] = row[A][t-1] 1 or so.
While the current code using iterators, scipy's zeros function, and datetime may work, it can be improved.
Why not grow a DataFrame row-wise?
Growing a DataFrame row-wise is generally not recommended for the following reasons:
-
Computational cost: Appending to a list and creating a DataFrame in one go is less computationally intensive than creating an empty DataFrame and appending to it over and over again.
-
Memory usage: Lists take up less memory and are a lighter data structure to work with than DataFrames, making them more efficient for appending and removing.
-
Data type inference: If you append to a DataFrame, you may end up with object columns, which can hinder pandas' performance. Lists, on the other hand, allow dtypes to be automatically inferred.
-
Index management: A RangeIndex is automatically created for your data when you create a DataFrame from a list, which saves you the hassle of managing the index yourself.
The recommended approach: Accumulate data in a list
Instead of growing a DataFrame row-wise, it is better to accumulate the data in a list and then initialize a DataFrame using pd.DataFrame(data). This approach offers the following advantages:
-
Efficiency: It is more computationally efficient and requires less memory.
-
Flexibility: Lists can be converted to both list-of-lists and list-of-dicts formats, which are accepted by pd.DataFrame.
-
Convenience: It handles index management and data type inference automatically.
Alternatives to consider
While accumulating data in a list is the preferred approach, there are two worse alternatives to avoid:
-
Append or concat inside a loop: This is inefficient and error-prone because it repeatedly reallocates memory and may lead to object columns.
-
Creating an empty DataFrame of NaNs: This approach also creates object columns and requires manual index management.
Conclusion
To effectively fill a DataFrame with values, it is best to accumulate the data in a list and then initialize the DataFrame using pd.DataFrame(data). This method is efficient, flexible, and convenient, making it the preferred approach for working with pandas DataFrames.
The above is the detailed content of How to Efficiently Fill a Pandas DataFrame Iteratively?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn