


Why Does Argument Dependent Lookup (ADL) Fail with Explicitly Instantiated Function Templates?
ADL and Function Template Lookup
Function templates play a significant role in C programming, enabling code reuse and generic functionality. However, a subtle limitation arises when using Argument Dependent Lookup (ADL) to locate function templates in specific namespaces.
The issue manifests itself when attempting to call a function template with explicit template arguments, as illustrated in the following code snippet:
namespace ns { struct foo {}; template<int i> void frob(foo const&) {} void non_template(foo const&) {} } int main() { ns::foo f; non_template(f); // This is fine. frob(f); // This is not. }</int>
The reason behind this failure stems from a specific clause in the C Standard. As per C Standard 03 14.8.1.6, ADL does not apply when calling function templates with explicit template arguments unless a function template with that name is visible at the point of the call.
In the example above, there is no function template named frob visible in the current scope when frob(f); is called. Therefore, ADL is not triggered, and the compiler fails to find the desired function template.
To circumvent this limitation, one can explicitly specify the namespace before the function template, as shown below:
ns::frob(f);
In this case, ADL is not necessary since the function template is directly invoked from the containing namespace.
The following code example further illustrates the behavior of ADL with function templates:
namespace A { struct B { }; template<int x> void f(B); } namespace C { template<class t> void f(T t); } void g(A::B b) { f(b); //ill-formed: not a function call A::f(b); //well-formed C::f(b); //ill-formed; argument dependent lookup // applies only to unqualified names using C::f; f(b); //well-formed because C::f is visible; then // A::f is found by argument dependent lookup }</class></int>
In this example, the function template f is defined in both namespaces A and C. When the call f(b); is made, the compiler first checks the current scope and finds no visible f template. ADL is then applied, but since f is an unqualified name, it cannot find function templates in other namespaces.
To call the template from namespace C, one must explicitly qualify the name with C::f or using directive as demonstrated in the code.
The above is the detailed content of Why Does Argument Dependent Lookup (ADL) Fail with Explicitly Instantiated Function Templates?. For more information, please follow other related articles on the PHP Chinese website!
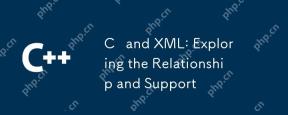
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
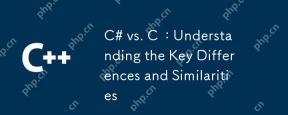
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
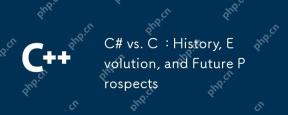
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
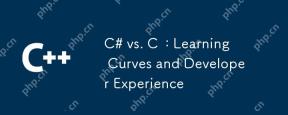
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
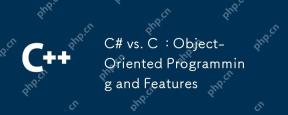
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
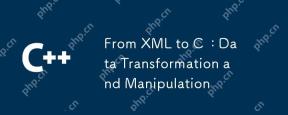
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
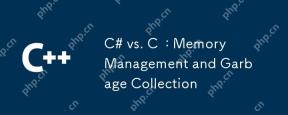
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
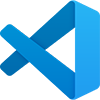
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software