


How to Retrieve Realtime Output and Print Processed Information from a Shell Command in Golang
In this guide, we'll focus on a specific scenario where we execute a shell command that produces output in real time, such as ffmpeg. We aim to retrieve this output steadily and print processed information based on the data it provides.
Let's break down the original code and explain the issue:
package main import ( "bufio" "fmt" "io" "os" "os/exec" "strings" ) func main() { // ... stdout, _ := cmd.StdoutPipe() cmd.Start() go print(stdout) cmd.Wait() } func print(stdout io.ReadCloser) { // ... }
The issue lies in assuming the output will be sent to stdout, but in reality, ffmpeg sends diagnostic messages to stderr instead. To capture the desired output, we need to pipe the stderr stream. Here's a revised code that addresses this:
func main() { // ... stderr, _ := cmd.StderrPipe() cmd.Start() scanner := bufio.NewScanner(stderr) scanner.Split(bufio.ScanWords) for scanner.Scan() { m := scanner.Text() fmt.Println(m) } cmd.Wait() }
Now, the code reads from the stderr pipe and prints the words it receives. This ensures that we capture and display the diagnostic messages generated by ffmpeg.
For instance, if ffmpeg's progress bar looks like this:
frame= 101 fps=0.0 q=28.0 size= 91kB time=00:00:04.13 bitrate= 181.2kbits/ frame= 169 fps=168 q=28.0 size= 227kB time=00:00:06.82 bitrate= 272.6kbits/ frame= 231 fps=153 q=28.0 size= 348kB time=00:00:09.31 bitrate= 306.3kbits/ frame= 282 fps=140 q=28.0 size= 499kB time=00:00:11.33 bitrate= 360.8kbits/
Our code will capture and print these words line by line:
frame= 101 fps= 0.0 q= 28.0 size= 91kB time= 00:00:04.13 bitrate= 181.2kbits/ ... (and so on)
You can then use these words to parse the relevant information and update your progress accordingly.
The above is the detailed content of How to Capture and Print Realtime Output from a Shell Command's Standard Error in Golang?. For more information, please follow other related articles on the PHP Chinese website!
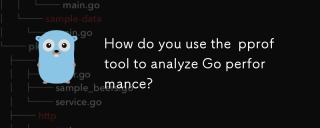
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
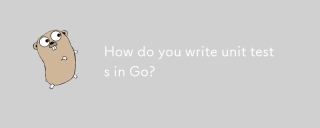
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
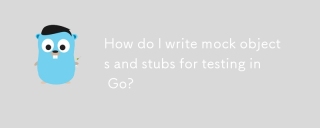
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
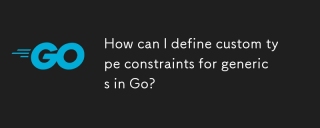
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
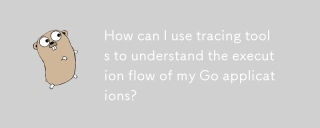
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
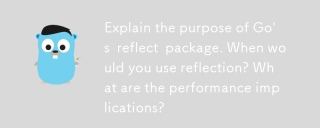
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
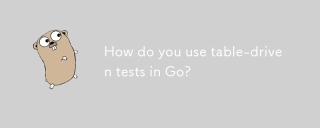
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
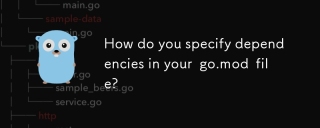
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
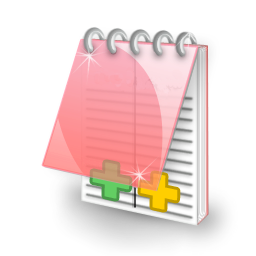
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
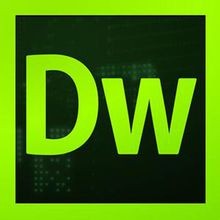
Dreamweaver CS6
Visual web development tools
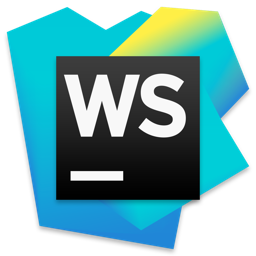
WebStorm Mac version
Useful JavaScript development tools
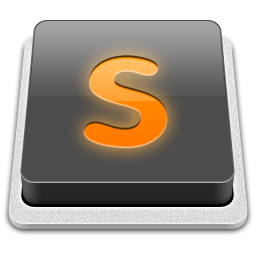
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
