Modifying Collections During Iteration: A Comprehensive Guide
To effectively modify collections during iteration to avoid errors like ConcurrentModificationException, there are several strategies to consider:
Collect and Remove
This method involves collecting objects to be removed during an enhanced for loop and then removing them after iteration completes. This technique is particularly useful in scenarios where deletion is the primary goal:
List<book> books = new ArrayList(); ISBN isbn = new ISBN("0-201-63361-2"); List<book> found = new ArrayList(); for (Book book : books) { if (book.getIsbn().equals(isbn)) { found.add(book); } } books.removeAll(found);</book></book>
Using ListIterator
ListIterator provides support for both removal and addition of items during iteration. This makes it a suitable choice when modifying lists:
List<book> books = new ArrayList(); ISBN isbn = new ISBN("0-201-63361-2"); ListIterator<book> iter = books.listIterator(); while (iter.hasNext()) { if (iter.next().getIsbn().equals(isbn)) { iter.remove(); } }</book></book>
JDK >= 8
Java 8 introduces additional methods for collection modification:
- removeIf: This method removes elements matching a specified predicate from the collection:
List<book> books = new ArrayList(); ISBN isbn = new ISBN("0-201-63361-2"); books.removeIf(book -> book.getIsbn().equals(isbn));</book>
- Stream API: The stream API allows for filtering and removing elements using pipelines:
List<book> books = new ArrayList(); ISBN isbn = new ISBN("0-201-63361-2"); List<book> filtered = books.stream() .filter(book -> book.getIsbn().equals(isbn)) .collect(Collectors.toList());</book></book>
Sublist or Subset
For sorted lists, removing consecutive elements can be done efficiently using sublists:
List<book> books = new ArrayList(); books.subList(0, 5).clear();</book>
Considerations
The choice of modification method depends on the specific scenario and collection type. Here are some key considerations:
- The "collect and remove" technique is versatile and works with any collection.
- The ListIterator approach is efficient but only applicable to lists with supporting implementations.
- The Iterator approach supports removal but may not be universally available.
- JDK 8 methods offer efficient filtering and removal but require transferring to a new collection.
- Sublists allow for bulk removal of consecutive elements in sorted lists.
The above is the detailed content of How Can I Safely Modify Collections While Iterating in Java?. For more information, please follow other related articles on the PHP Chinese website!
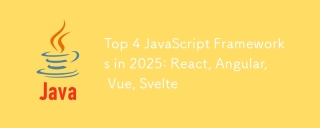
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
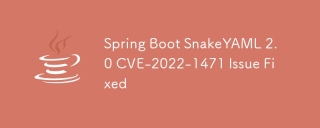
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
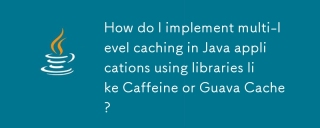
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
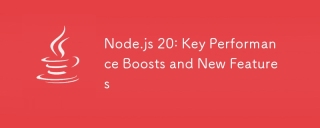
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
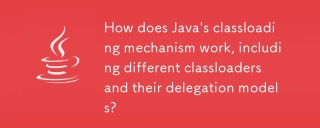
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
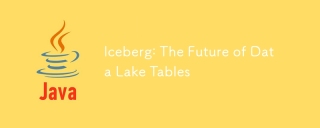
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
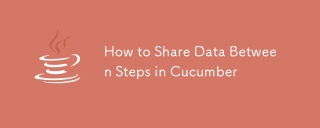
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
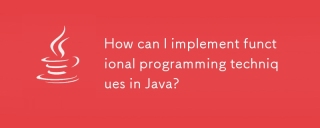
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
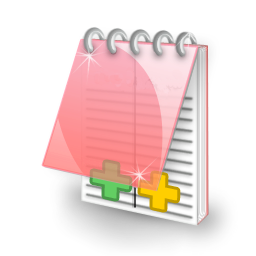
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
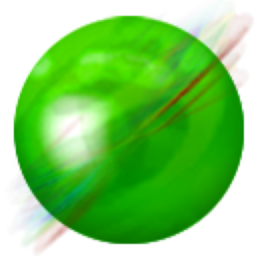
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
