Uploading Images to a MySQL Database Using PHP
Inserting images into a MySQL database using PHP requires several steps. Let's address the issues in the code you provided:
1. Database Table Structure:
Ensure that your database table has a column defined as BLOB or MEDIUMBLOB type to store the image data.
2. Prepared Statements:
Instead of using the deprecated mysql_query() function, switch to prepared statements using PDO or MySQLi. Prepared statements prevent SQL injection vulnerabilities.
3. Proper Image Handling:
The current code retrieves the image data directly from the temporary file. This is a security risk. Instead, use file_get_contents() with the FILE_BINARY option to ensure that the binary content is properly handled.
4. Sanitization:
Sanitize the image data using addslashes() or mysqli_real_escape_string() to prevent SQL injection.
5. Correct Insert Query:
The insert query should match the table structure and sanitize the data accordingly. Here's a corrected version:
$stmt = $mysqli->prepare("INSERT INTO product_images (id, image, image_name) VALUES (?, ?, ?)"); $stmt->bind_param("sis", 1, $image, $image_name); $image = file_get_contents($_FILES['image']['tmp_name'], FILE_BINARY); $image_name = addslashes($_FILES['image']['name']); if ($stmt->execute()) { echo "Image uploaded successfully"; } else { echo "Error: " . $mysqli->error; }
6. HTML Form:
The HTML form should use the enctype="multipart/form-data" attribute, which allows binary data to be transmitted in the request. Additionally, use a proper closing tag for the
The above is the detailed content of How to Properly Upload Images to a MySQL Database Using PHP?. For more information, please follow other related articles on the PHP Chinese website!
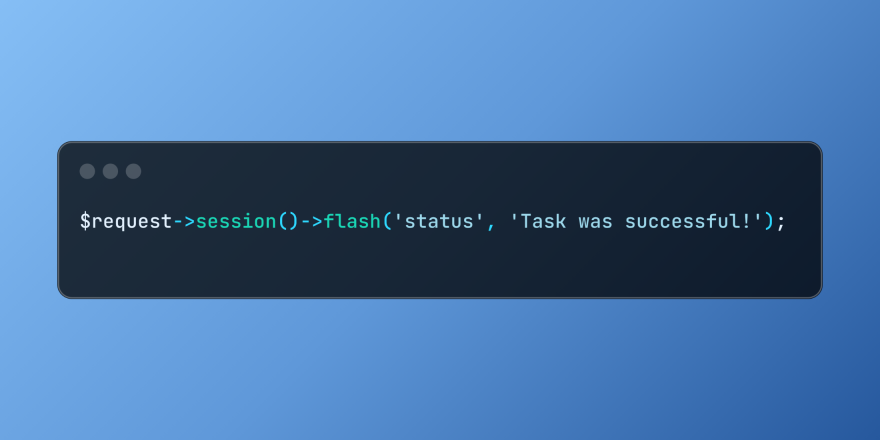
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
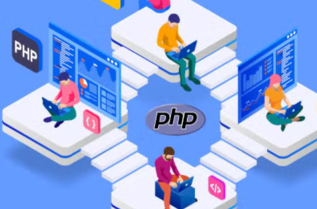
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
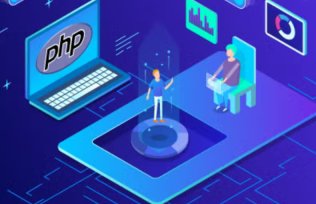
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
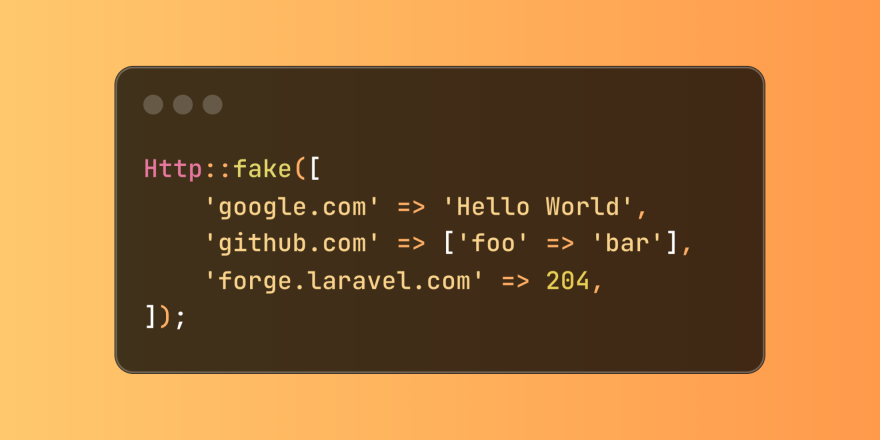
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
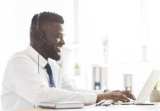
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
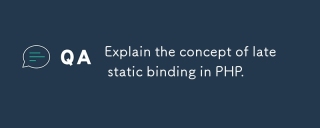
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
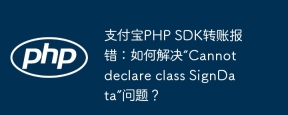
Alipay PHP...
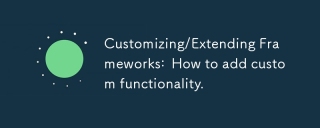
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),