


Compiler Optimization and Undefined Behavior: Does C Allow Certain Assumptions About Bools?
Introduction
This article examines whether the C standard permits compilers to assume certain numerical representations for bools and whether such assumptions can lead to consequences such as program crashes.
The Issue
A programmer encountered a program crash while using an uninitialized bool value in a function that serialized a bool into a string. Surprisingly, the crash occurred only on a specific platform using a specific compiler with optimization enabled.
The problematic code:
void Serialize(bool boolValue) { const char* whichString = boolValue ? "true" : "false"; const size_t len = strlen(whichString); memcpy(destBuffer, whichString, len); }
When the code is executed with Clang 5.0.0 and optimization (-O2), it may crash. This behavior arises due to the optimizer's deduction that the strings "true" and "false" differ only in length by 1. Instead of calculating the actual length, it uses the value of boolValue, assuming it is either 0 or 1.
const size_t len = strlen(whichString); // original code const size_t len = 5 - boolValue; // clang optimization
Question: Standard Considerations
The article poses the question: Does the C standard allow a compiler to assume that a bool can only have an internal numerical representation of '0' or '1' and use it in such a way? Or is this a case of implementation-defined behavior where the implementation has assumed all its bools will only ever contain 0 or 1, and any other value is undefined behavior territory?
Answer: Standard Conformity
According to the author, ISO C allows (but doesn't require) implementations to make this choice. ISO C leaves it unspecified what the internal representation of a bool is, allowing implementations to make their own assumptions.
Compiler Optimization Behavior
System V ABI: For platforms using the System V ABI, which is commonly used on x86-64 systems, a bool argument passed to a function is represented by the bit-patterns: 0 = false and 1 = true in the low 8 bits of the register. In memory, bool is a 1-byte type that must have an integer value of 0 or 1.
This ABI decision allows the compiler to take advantage of optimizations, such as assuming 0 or 1 for bool and performing bitwise operations instead of expensive type conversions. In the example provided, the optimizer has exploited this behavior to optimize strlen(whichString) to 5U - boolValue.
Other Implementations and Assumptions:
While the System V ABI is widely used, other implementations could make different assumptions. For example, they could consider 0 = false and any non-zero value = true. In such a scenario, the compiler might not generate code that crashes for uninitialized bool values, but it could still be considered undefined behavior.
The Dangers of Program Crashes
While the C standard allows such optimizations, it's important to note that programs encountering undefined behavior are considered totally undefined for their entire existence. This means that a crash can occur even if the undefined behavior is encountered in a function that is never actually called.
Best Practices and Avoiding Undefined Behavior
Compilers are becoming increasingly aggressive in optimizing code, assuming behaviors based on their internal understanding of the implementation. It's crucial for programmers to avoid relying on implementation assumptions and ensure that their code is valid C without assuming it will behave like a portable assembly language.
To avoid problems, programmers should follow these best practices:
- Use the -Wall compiler flag to enable warnings.
- Fix all warnings generated by your compiler.
- Be aware that assumptions about uninitialized variables can lead to program crashes.
- Consider using tools like Address Sanitizer and Memory Sanitizer to detect usage of uninitialized values and potential undefined behavior.
The above is the detailed content of Can C Compilers Assume a Boolean's Numerical Representation is Only 0 or 1, and Does This Lead to Undefined Behavior?. For more information, please follow other related articles on the PHP Chinese website!
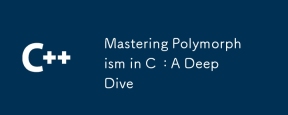
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
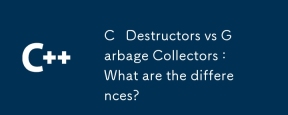
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
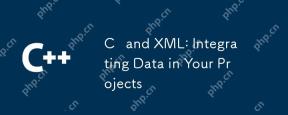
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
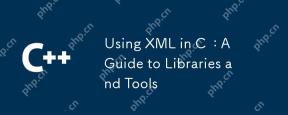
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
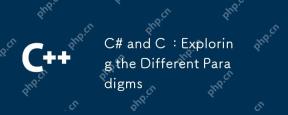
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
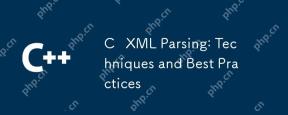
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
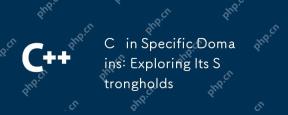
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
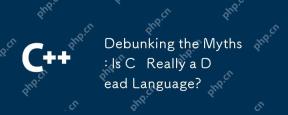
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
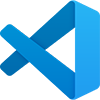
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
