Understanding the Single Responsibility Principle in JavaScript
When writing clean, maintainable code, one of the most important principles to follow is the Single Responsibility Principle (SRP). It’s one of the five SOLID principles in software development and ensures that your code is easier to read, test, and modify.
What is the Single Responsibility Principle?
The Single Responsibility Principle according to Robert C.Martin states that:
A class or function should have one and only one reason to change.
In simpler terms, each unit of your code (be it a function, class, or module) should be responsible for doing one thing and doing it well. When responsibilities are separated, changes in one area of your code won't unexpectedly affect others, reducing the risk of bugs and making your application easier to maintain and test.
Why is SRP Important?
Without SRP, you might face problems like:
- Tangled Dependencies: When a function or class has multiple responsibilities, making a change to one can inadvertently break another.
- Reduced Testability: Testing becomes harder when a unit of code does too much, as you’d need to mock unrelated dependencies. Poor Readability: Large, multi-purpose functions or classes are harder to understand, especially for new developers joining the project.
- Difficult Maintenance: The more responsibilities a unit has, the more effort it takes to isolate and fix bugs or add new features.
Applying SRP in JavaScript
Let’s look at some practical examples of applying SRP in JavaScript.
Example 1: Refactoring a Function
Without SRP
function handleUserLogin(userData) { // Validate user data if (!userData.email || !userData.password) { logger.error("Invalid user data"); return "Invalid input"; } // Authenticate user const user = authenticate(userData.email, userData.password); if (!user) { console.error("Authentication failed"); return "Authentication failed"; } // Log success console.info("User logged in successfully"); return user; }
This function does too much: validation, authentication, and logging. Each of these is a distinct responsibility.
With SRP
We can refactor it by breaking it into smaller, single-purpose functions:
function validateUserData(userData) { if (!userData.email || !userData.password) { throw new Error("Invalid user data"); } } function authenticateUser(email, password) { const user = authenticate(email, password); // Assume authenticate is defined elsewhere if (!user) { throw new Error("Authentication failed"); } return user; } function handleUserLogin(userData, logger) { try { validateUserData(userData); const user = authenticateUser(userData.email, userData.password); logger.info("User logged in successfully"); return user; } catch (error) { logger.error(error.message); return error.message; } }
Now, each function has a single responsibility, making it easier to test and modify.
Example 2: Refactoring a Class
Without SRP
A class managing multiple concerns:
class UserManager { constructor(db, logger) { this.db = db; this.logger = logger; } createUser(user) { // Save user to DB this.db.save(user); this.logger.info("User created"); } sendNotification(user) { // Send email emailService.send(`Welcome, ${user.name}!`); this.logger.info("Welcome email sent"); } }
Here, UserManager handles user creation, logging, and sending emails—too many responsibilities.
With SRP
Refactor by delegating responsibilities to other classes or modules:
class UserService { constructor(db) { this.db = db; } createUser(user) { this.db.save(user); } } class NotificationService { sendWelcomeEmail(user) { emailService.send(`Welcome, ${user.name}!`); } } class UserManager { constructor(userService, notificationService, logger) { this.userService = userService; this.notificationService = notificationService; this.logger = logger; } createUser(user) { this.userService.createUser(user); this.notificationService.sendWelcomeEmail(user); this.logger.info("User created and welcome email sent"); } }
Each class now focuses on a single concern: persistence, notification, or logging.
Tips for Following SRP
- Keep Functions Short: Aim for functions that are 5–20 lines long and serve one purpose.
- Use Descriptive Names: A good function or class name reflects its responsibility.
- Refactor Often: If a function feels too big or hard to test, split it into smaller functions.
- Group Related Logic: Use modules or classes to group related responsibilities, but avoid mixing unrelated ones.
Conclusion
The Single Responsibility Principle is a cornerstone of clean code. By ensuring that every function, class, or module has only one reason to change, you make your JavaScript code more modular, easier to test, and simpler to maintain.
Start small—pick one messy function or class in your current project and refactor it using SRP. Over time, these small changes will lead to a significant improvement in your codebase.
The above is the detailed content of Single Responsibility Principle in Javascript. For more information, please follow other related articles on the PHP Chinese website!
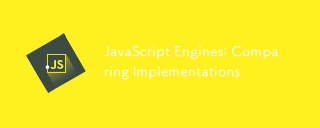
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
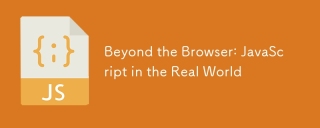
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
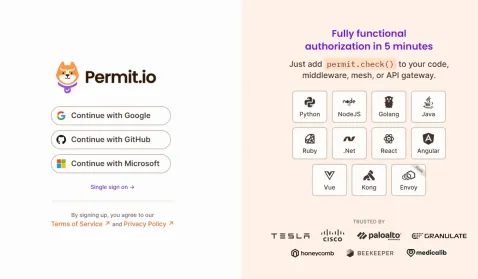
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
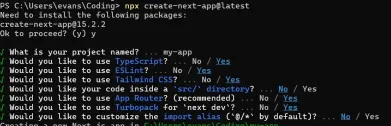
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
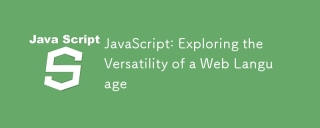
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
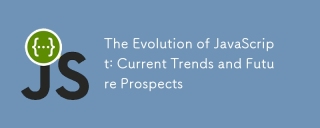
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
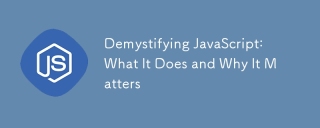
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
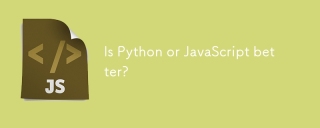
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
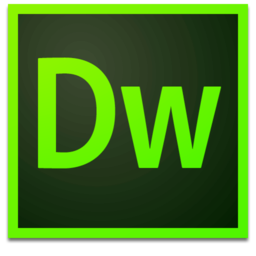
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.