Converting a Slice to an Array in Go without Copying
When working with slices and arrays in Go, it can be necessary to convert between the two types. A common scenario is converting a slice into an array without making a copy. This can be achieved using a few different methods.
Using the copy function
The copy function allows you to copy elements from one slice to another. However, it can only copy between slices, not between slices and arrays. To work around this, you can use a trick:
varLead := Lead{} copy(varLead.Magic[:], someSlice[0:4])
In this code, varLead.Magic is an array of size 4, while someSlice is a slice. By using the [:] syntax on varLead.Magic, we create a slice header that points to the underlying array. The copy function then copies the elements from someSlice[0:4] into this slice header, effectively converting it to an array without making a copy.
Using a for loop
Another option is to use a for loop to manually copy the elements from the slice to the array:
for index, b := range someSlice { varLead.Magic[index] = b }
This code iterates over the elements in someSlice and assigns each element to the corresponding index in varLead.Magic. It is a simple and straightforward way to convert a slice to an array without making a copy.
Using literals
Finally, you can also use literals to create an array from a slice:
varLead.Magic = [4]byte{someSlice[0], someSlice[1], someSlice[2], someSlice[3]}
This code explicitly creates an array of size 4 and assigns the first four elements of someSlice to it. It is the most compact and readable way to convert a slice to an array, but it can become cumbersome if the array has a large number of elements.
The above is the detailed content of How to Convert a Go Slice to an Array Without Copying?. For more information, please follow other related articles on the PHP Chinese website!
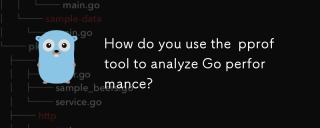
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
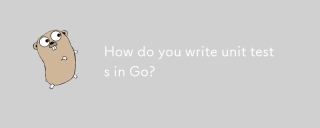
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
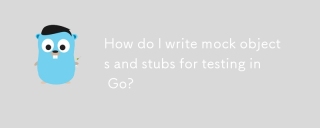
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
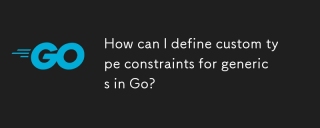
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
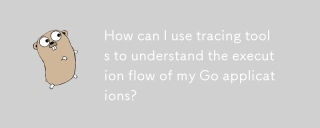
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
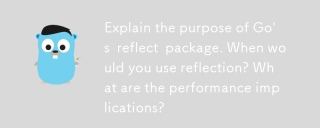
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
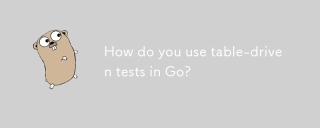
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
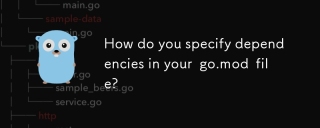
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
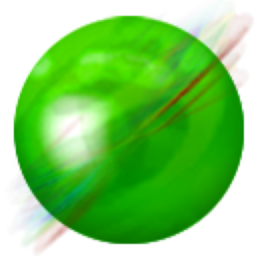
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
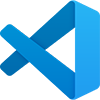
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
