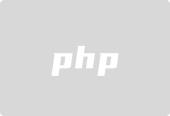
Deoptimizing a program for the pipeline in Intel Sandybridge-family CPUs
The goal of this assignment is to modify a given program to make it run slower, while maintaining the same algorithm. This is to gain a deeper understanding of how the Intel i7 pipeline operates and how instruction paths can be re-ordered to introduce hazards.
Program Overview
The program is a Monte-Carlo simulation that prices European vanilla call and put options. It uses the Box-Muller algorithm to generate Gaussian random numbers and performs a large number of simulations to estimate the option prices.
Diabolical Incompetence
The objective is to intentionally create incompetent code that will slow down the program's execution. Here are some ideas that justify this approach with "diabolical incompetence":
False Sharing
- Create shared data structures that are accessed by multiple threads, but are not properly synchronized. This can lead to cache-line ping-ponging and memory-order mis-speculation pipeline clears.
Store-Forwarding Stalls
- Avoid using the "-" operator for floating-point variables. Instead, XOR the high byte with 0x80 to flip the sign bit, causing store-forwarding stalls.
Excessive Time Measurement
- Time each iteration of the main loop with a heavy operation like CPUID/RDTSC, which serializes instructions and stalls the pipeline.
Unfavorable Mathematical Operations
- Replace multiplications by constants with divisions by their reciprocal ("for ease of reading"). Division is slower and not fully pipelined.
Inefficient Vectorization
- Vectorize the multiply/sqrt operations with AVX, but fail to use vzeroupper before calling scalar math-library functions, causing AVX<>SSE transition stalls.
Data Structures
- Store the RNG output in a linked list or in arrays that are traversed out of order. Do the same for the result of each iteration and sum at the end. This introduces pointer-chasing loads and defeats cache locality.
Multi-threading Misuse
- Multi-thread the program but force both threads to share the same loop counter (with atomic increments) to create false sharing and contention. This also introduces unnecessary overhead from the atomic operations.
Other Suggestions
- Introduce unpredictable branches to create mispredictions and pipeline flushes.
- Use diabolically incompetent justifications to increase the length of loop-carried dependency chains.
- Introduce non-contiguous memory access patterns to minimize cache utilization.
-
For extra credit: Write your own square root algorithm to replace the one provided in the assignment.
Impact of Modifications
These modifications are expected to significantly slow down the program's execution by introducing pipeline stalls, cache misses, and other performance bottlenecks. The assignment encourages creativity and a willingness to explore different methods of pessimizing the code while maintaining the original algorithm.
The above is the detailed content of How Can We Deoptimize a Monte Carlo Simulation to Expose Intel Sandybridge Pipeline Bottlenecks?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn