Uploading Images into MySQL Database Using PHP Code
This guide addresses the common issue faced when attempting to save images into a MySQL database using PHP code. The issue is that the code doesn't generate any error messages but also fails to insert image data into the database. Following are the steps to resolve the issue:
1. Ensure the Image Column is BLOB Type:
Verify that the column designed to store images in the MySQL table is of the BLOB type. BLOB (Binary Large Object) is used to store binary data like images.
2. Escape All Data for SQL Injection Prevention:
Data inserted into the database should be escaped to prevent SQL Injection vulnerabilities. Use PHP's addslashes() function to escape the image data before inserting it.
3. Use Correct SQL Syntax:
The SQL query used to insert the image data should follow the correct syntax. Ensure to specify the correct column names and data types.
4. Use Modern Database Drivers:
The example code provided uses deprecated MySQL functions. It's recommended to use modern database drivers like PDO or MySQLi for improved security and efficiency.
5. Follow HTML Form Standards:
The HTML form should follow web standards. The correct form syntax for uploading images is:
Example Using PDO:
$conn = new PDO('mysql:host=<host>;dbname=<database>;charset=utf8', '<username>', '<password>'); $image = addslashes(file_get_contents($_FILES['image']['tmp_name'])); $sql = "INSERT INTO product_images (id, image, image_name) VALUES (?, ?, ?)"; $stmt = $conn->prepare($sql); $stmt->execute([1, $image, $_FILES['image']['name']]);</password></username></database></host>
By following these steps, you can successfully upload images into your MySQL database and prevent any potential errors.
The above is the detailed content of How to Successfully Upload Images to a MySQL Database Using PHP?. For more information, please follow other related articles on the PHP Chinese website!
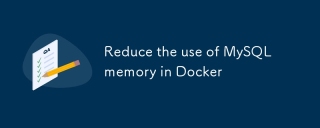
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
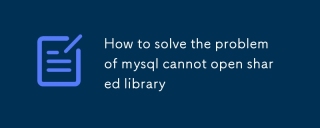
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
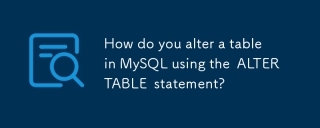
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
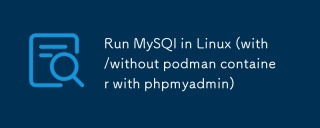
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
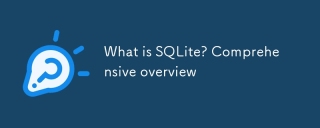
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
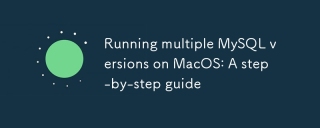
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
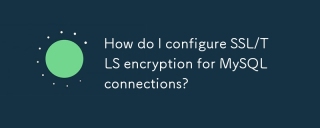
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
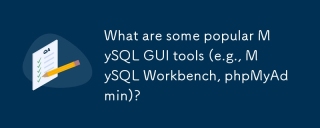
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
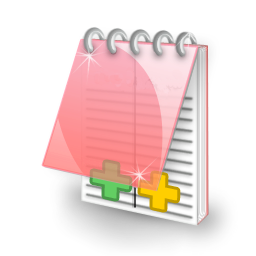
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
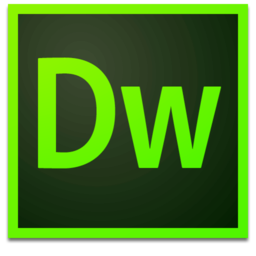
Dreamweaver Mac version
Visual web development tools
