Understanding Stack and Heap Usage in C
Managing memory effectively is crucial in C programming. When determining where to store variables, one must decide between the stack and the heap.
Stack vs. Heap: A Matter of Lifespan
Contrary to popular belief, performance is not the primary factor in selecting between the stack and heap. The key difference lies in the lifespan of variables.
- Stack: Stores local variables within a function that are accessible only within that function. These variables are automatically destroyed upon function return.
-
Heap: Stores objects, rarely used variables, and large data structures that need to outlast their declaring function.
Specific Examples for Clarity
Consider the following code snippet:
class Thingy; Thingy* foo( ) { int a; // Stack-allocated integer Thingy B; // Stack-allocated Thingy object Thingy *pointerToB = &B; // Pointer to stack-allocated object Thingy *pointerToC = new Thingy(); // Heap-allocated Thingy object // Safe: Heap-allocated Thingy outlives foo() return pointerToC; // Unsafe: Stack-allocated Thingy will be destroyed upon foo() return return pointerToB; }
In this example:
- a and B are stored on the stack because they are local to foo().
- pointerToB points to a stack-allocated object.
- pointerToC points to a heap-allocated object that outlives foo().
-
Attempting to return pointerToB can lead to a crash, as the stack-allocated object it points to will be destroyed.
Critical Distinction: Computer Reality vs. Language Abstraction
Understanding the stack concept requires a deeper dive into the underlying machine. "Heap" and "stack" are compiler inventions, and the computer's memory is simply an array of addresses. By examining concepts such as the call stack and calling convention, one can grasp how the machine executes functions and manages memory.
The above is the detailed content of Stack vs. Heap in C : When Should I Use Each?. For more information, please follow other related articles on the PHP Chinese website!
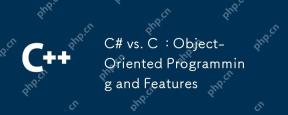
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
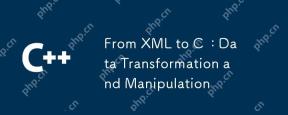
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
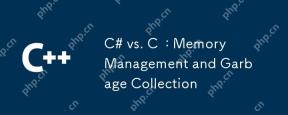
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
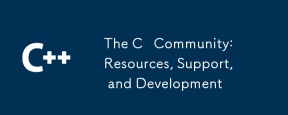
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
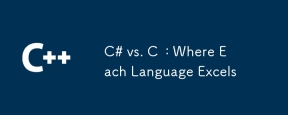
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
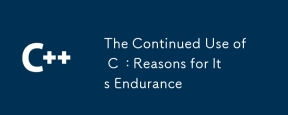
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
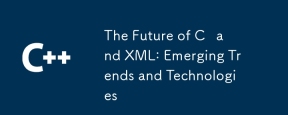
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
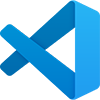
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
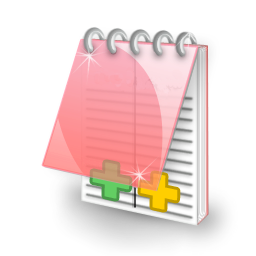
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function