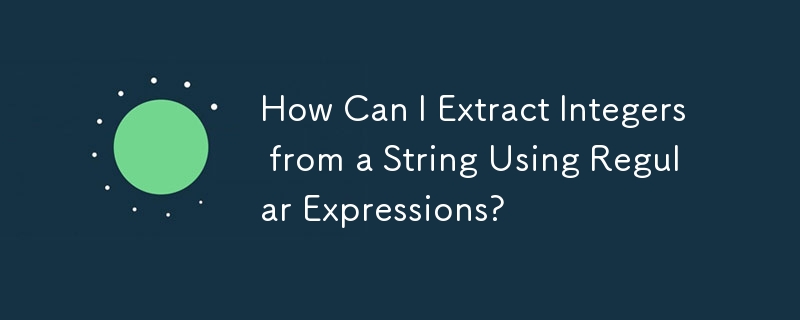
Extracting Integers from a Textual String using Regular Expressions
When working with data that combines text and numerical values, it becomes necessary to extract those numbers and convert them into a usable format. Regular expressions provide a powerful means to achieve this extraction from a given input string.
To extract all the integers from a string and store them in an array of integers, follow these steps:
-
Define a Regular Expression: Create a regular expression pattern that matches numerical patterns in the string. Use Pattern p = Pattern.compile("\d "); to detect one or more consecutive digits. Note that this pattern only matches non-negative numbers.
-
Obtain a Matcher: Create a Matcher object using the Pattern. Use Matcher m = p.matcher(line); to associate the pattern with the input string.
-
Iterate through Matches: Use a loop to iterate through the matches found by the Matcher. In each iteration, use m.find() to find a match and m.group() to extract the matched number.
-
Parse Integers (Optional): If necessary, parse the extracted numbers into integers using Integer.parseInt(m.group()).
-
Store in an Array: Create an array to store the extracted integers and add the parsed integers to the array in each iteration.
Example:
Pattern p = Pattern.compile("-?\d+");
Matcher m = p.matcher("There are more than -2 and less than 12 numbers here");
while (m.find()) {
System.out.println(m.group());
}
Output:
-2
12
This regular expression pattern will match both positive and negative numbers, allowing for more robust number extraction.
The above is the detailed content of How Can I Extract Integers from a String Using Regular Expressions?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn