


How to Efficiently Merge JavaScript Objects with Matching Keys and Sum Their Values?
Merging Objects with Matching Properties in JavaScript Arrays
Consider the following array of objects:
objArr = [ {key:"Mon Sep 23 2013 00:00:00 GMT-0400", val:42}, {key:"Mon Sep 24 2013 00:00:00 GMT-0400", val:78}, {key:"Mon Sep 25 2013 00:00:00 GMT-0400", val:23}, {key:"Mon Sep 23 2013 00:00:00 GMT-0400", val:54}, // <p>Our goal is to merge duplicate keys and sum their respective values, resulting in:</p><pre class="brush:php;toolbar:false">reducedObjArr = [ {key:"Mon Sep 23 2013 00:00:00 GMT-0400", val:96}, {key:"Mon Sep 24 2013 00:00:00 GMT-0400", val:78}, {key:"Mon Sep 25 2013 00:00:00 GMT-0400", val:23} ]
While an initial attempt might involve iterating and pushing values, a more efficient approach involves leveraging JavaScript's built-in functions:
// Use a Map to count values with the same key const counts = objArr.reduce((prev, curr) => { let count = prev.get(curr.key) || 0; prev.set(curr.key, curr.val + count); return prev; }, new Map()); // Convert the Map back to an array of objects const reducedObjArr = [...counts].map(([key, value]) => { return {key, value}; });
This method reduces the array to a Map where keys represent duplicate key values and values represent their sums. The Map is then converted back to an array of objects for readability.
The above is the detailed content of How to Efficiently Merge JavaScript Objects with Matching Keys and Sum Their Values?. For more information, please follow other related articles on the PHP Chinese website!
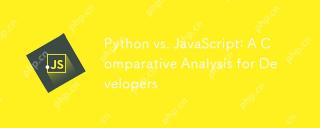
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
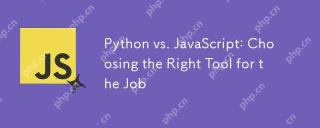
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
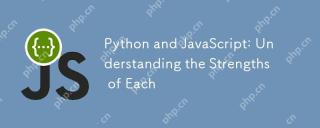
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
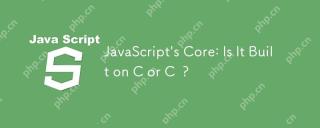
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
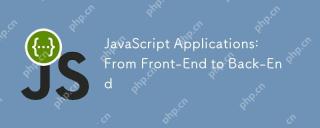
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
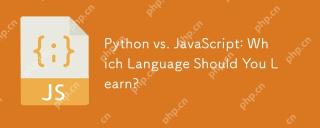
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
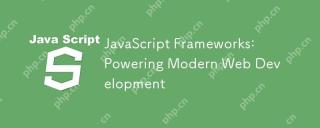
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
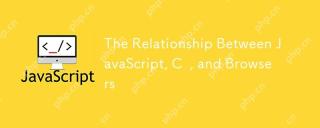
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
