


How to Efficiently Convert CGO Arrays to Go Slices: Safe vs. Unsafe Methods?
Unconventional Casting: Converting CGO Arrays to Go Slices
When working with CGO in Go, one may encounter the need to cast a CGO array into a Go slice. Traditionally, this involves a manual conversion by iterating over the array and casting each element individually.
However, there exist more efficient and unconventional approaches to this task.
Safe and Straightforward Method:
The safer and more recommended approach is to use a for loop to manually cast each element of the CGO array:
c := [6]C.double{1, 2, 3, 4, 5, 6} fs := make([]float64, len(c)) for i := range c { fs[i] = float64(c[i]) }
This method guarantees type safety and portability.
Unconventional but Efficient Method:
For speed and efficiency, a more daring approach can be employed, known unsafe type casting:
c := [6]C.double{1, 2, 3, 4, 5, 6} cfa := (*[6]float64)(unsafe.Pointer(&c)) cfs := cfa[:]
This technique relies on the assumption that C.double and float64 share the same underlying type. It involves taking a pointer to the CGO array, casting it unsafely to a pointer to a float64 array of the same size, and finally taking a slice of that array.
Caveats and Considerations:
While the unsafe conversion method may be faster, it comes with the caveat of potential undefined behavior and platform-dependency. It is important to ensure that the underlying types of C.double and float64 are indeed compatible before employing this approach. Additionally, it is critical to use this technique only when necessary and with utmost caution.
The above is the detailed content of How to Efficiently Convert CGO Arrays to Go Slices: Safe vs. Unsafe Methods?. For more information, please follow other related articles on the PHP Chinese website!
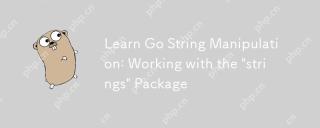
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
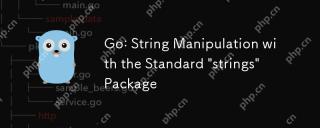
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
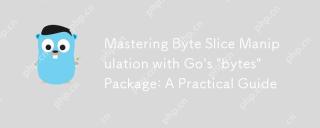
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
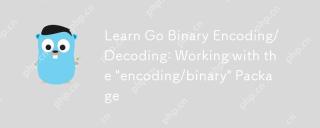
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
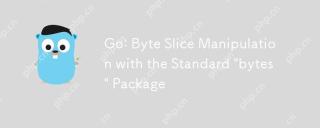
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
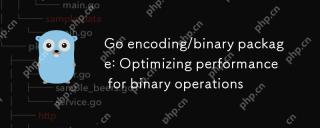
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
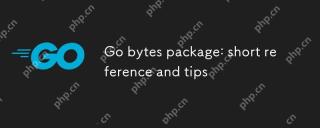
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
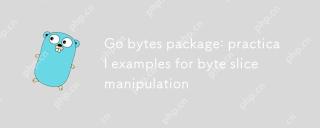
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
