Testing Go Functions with log.Fatal()
When writing Go functions that utilize log.Fatal(), testing them can pose a challenge due to its immediate termination of the program. To overcome this, we require a solution that allows us to test the desired logging behavior without halting the test execution.
One approach is to create a custom logger that overrides the default log.Fatal() function with one that records the log message without exiting the program. This custom logger can then be used within the test context, enabling the verification of expected log messages.
For example, suppose we have a function that logs a "Hello!" message and another that logs a "Goodbye!" message using log.Fatal().
package main import ( "log" ) func hello() { log.Print("Hello!") } func goodbye() { log.Fatal("Goodbye!") } func init() { log.SetFlags(0) } func main() { hello() goodbye() }
To test these functions, we can create a custom buffer logger:
package main import ( "bytes" "log" "testing" ) type BufferLogger struct { buf bytes.Buffer } func (l *BufferLogger) Println(v ...interface{}) { l.buf.WriteString(fmt.Sprintln(v...)) } func TestHello(t *testing.T) { l := &BufferLogger{} log.SetOutput(l) hello() wantMsg := "Hello!\n" msg := l.buf.String() if msg != wantMsg { t.Errorf("%#v, wanted %#v", msg, wantMsg) } }
In this custom BufferLogger, we redirect all log output to a buffer instead of the default console. This allows us to capture the log messages and compare them to the expected values within our test.
For the goodbye() function, we can create a similar test by overriding the Fatal() function in our custom logger to prevent the program from exiting:
func (l *BufferLogger) Fatalf(format string, v ...interface{}) { l.buf.WriteString(fmt.Sprintf(format, v...)) } func TestGoodbye(t *testing.T) { l := &BufferLogger{} log.SetOutput(l) goodbye() wantMsg := "Goodbye!\n" msg := l.buf.String() if msg != wantMsg { t.Errorf("%#v, wanted %#v", msg, wantMsg) } }
By overriding the log.Fatal() function, we can continue our test execution while still capturing the expected log message. This technique allows us to comprehensively test our functions that utilize log.Fatal() without encountering any premature program termination.
The above is the detailed content of How Can I Effectively Test Go Functions Using log.Fatal()?. For more information, please follow other related articles on the PHP Chinese website!
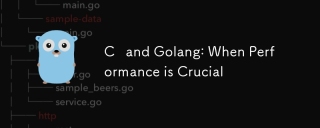
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
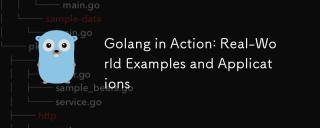
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
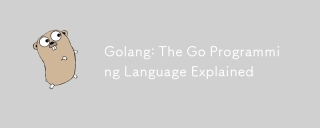
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
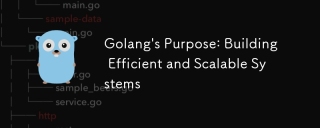
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
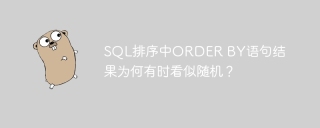
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
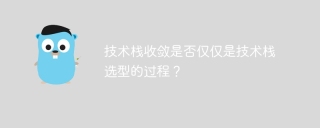
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
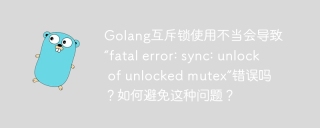
Golang ...
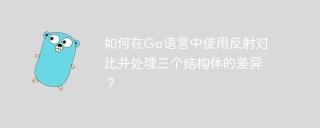
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
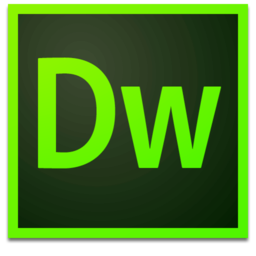
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.