


How can I dynamically update a Google Chart using AJAX based on user input from a dropdown menu?
Updating Google Charts Based on User Input via AJAX
Problem
A Google Chart is currently configured to fetch data from a specific table based on a GET request. The goal is to update this chart dynamically via AJAX based on a user's selection from a drop-down menu.
Analysis
The core issue arises from a lack of response from the chart to AJAX updates. A JSON format mismatch may be preventing Google from rendering the chart.
Solution
PHP Code (getdata.php):
<?php require("dbconnect.php"); $db = mysql_connect($server, $user_name, $password); mysql_select_db($database); $sqlQuery = "SELECT * FROM ".$_GET['q']." WHERE Date(Time + INTERVAL 10 HOUR) = Date(UTC_TIMESTAMP() + INTERVAL 10 HOUR)"; $sqlResult = mysql_query($sqlQuery); $rows = array(); $table = array(); // Define column labels $table['cols'] = array( array('label' => 'Time', 'type' => 'string'), array('label' => 'Wind_Speed', 'type' => 'number'), array('label' => 'Wind_Gust', 'type' => 'number') ); // Populate data rows while ($row = mysql_fetch_assoc($sqlResult)) { $temp = array(); $temp[] = array('v' => (string) $row['Time']); $temp[] = array('v' => (float) $row['Wind_Speed']); $temp[] = array('v' => (float) $row['Wind_Gust']); $rows[] = array('c' => $temp); } $table['rows'] = $rows; echo json_encode($table); ?>
HTML/Javascript:
<meta http-equiv="content-type" content="text/html; charset=utf-8"> <title>Wind Graph</title> <script src="http://www.google.com/jsapi"></script> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <script> google.load('visualization', '1', { callback: function () { // Add event listener to select element $("#users").change(drawChart); function drawChart() { $.ajax({ url: 'getdata.php', // Use value from select element data: 'q=' + $("#users").val(), dataType: 'json', success: function (responseText) { // Use response from PHP for data table var data = new google.visualization.DataTable(responseText); new google.visualization.LineChart(document.getElementById('visualization')). draw(data, { curveType: 'none', title: 'Wind Chart', titleTextStyle: { color: 'orange' }, interpolateNulls: 1 }); }, error: function(jqXHR, textStatus, errorThrown) { console.log(errorThrown + ': ' + textStatus); } }); } }, packages: ['corechart'] }); </script>
Key Differences:
- The getdata.php script now returns data in JSON format compatible with Google Charts.
- The hAxis and vAxis are specified only once in the chart options, instead of appearing twice.
- The async: false option is removed to prevent unexpected behavior.
- Inline event handlers are not used, and instead, a jQuery change listener is added.
The above is the detailed content of How can I dynamically update a Google Chart using AJAX based on user input from a dropdown menu?. For more information, please follow other related articles on the PHP Chinese website!
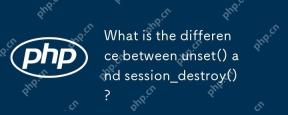
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
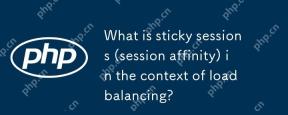
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
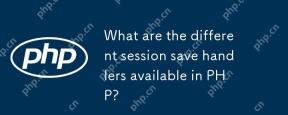
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
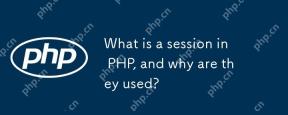
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
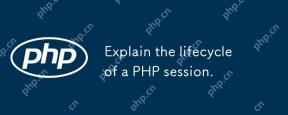
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
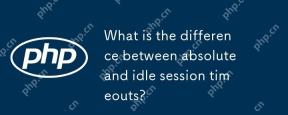
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
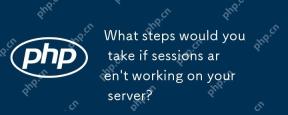
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
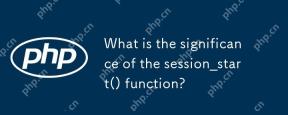
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
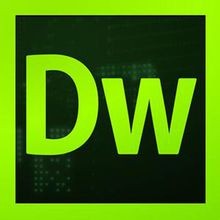
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
