


How to Implement a Timeout Function in Shell Scripting with a Predefined Duration?
Timeout Function with a Predefined Duration
In shell scripting, it's useful to have a mechanism to terminate long-running tasks to avoid excessive waiting. The challenge faced here is to wrap a function within a timeout script, returning False if it exceeds a specified time limit.
One approach is to set an asynchronous timer that triggers a False response after a pre-defined interval. Fortunately, such a solution is achievable using signal handlers in the signal library (available on UNIX-based systems).
The process involves creating a custom decorator (@timeout) that leverages signal.alarm() to set an alarm for the desired time interval. Within the decorated function, when the alarm expires, a TimeoutError exception is raised, effectively interrupting the operation.
To incorporate this solution into your code, save the following code as timeout.py and import it:
import errno import os import signal import functools class TimeoutError(Exception): pass def timeout(seconds=10, error_message=os.strerror(errno.ETIME)): def decorator(func): def _handle_timeout(signum, frame): raise TimeoutError(error_message) @functools.wraps(func) def wrapper(*args, **kwargs): signal.signal(signal.SIGALRM, _handle_timeout) signal.alarm(seconds) try: result = func(*args, **kwargs) finally: signal.alarm(0) return result return wrapper return decorator
Now, within your application code, annotate any potentially long-running function with the @timeout decorator. For example:
from timeout import timeout # Short timeout @timeout(5) def slow_function(): # ... # Default timeout @timeout def another_slow_function(): # ... # Customize timeout and error message @timeout(30, error_message="Task timed out") def yet_another_slow_function(): # ...
This approach ensures that if a function takes longer than the specified timeout interval, it will raise a TimeoutError and return False, allowing you to handle unexpected delays gracefully.
The above is the detailed content of How to Implement a Timeout Function in Shell Scripting with a Predefined Duration?. For more information, please follow other related articles on the PHP Chinese website!
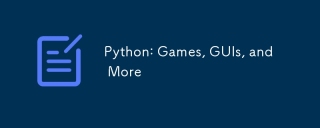
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
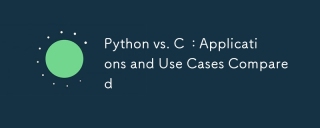
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
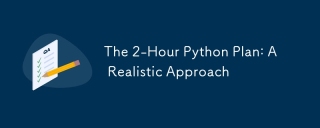
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
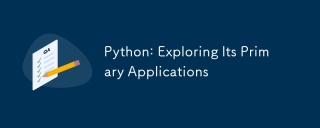
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
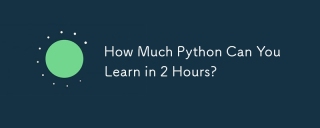
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
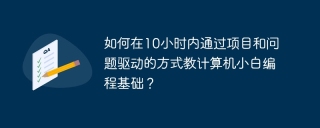
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
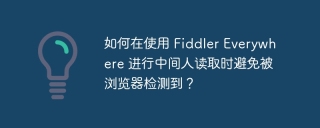
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
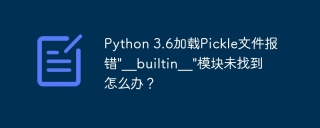
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
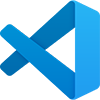
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version
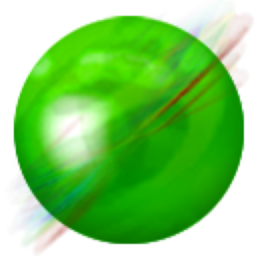
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor