Promisifying Native XHR: A Simplified Approach
In frontend applications, native promises offer a convenient way to handle asynchronous operations. However, incorporating them into native XHR requests can be challenging without relying on complex frameworks. This article aims to bridge this gap by providing a simplified guide on promisifying native XHR requests.
Understanding the Issue
Prior to promisifying XHR requests, it's essential to understand the typical callback-based approach. Here's an example of a basic XHR request using callbacks:
function makeXHRRequest(method, url, done) { var xhr = new XMLHttpRequest(); xhr.open(method, url); xhr.onload = function () { done(null, xhr.response); }; xhr.onerror = function () { done(xhr.response); }; xhr.send(); }
This approach works well for simple scenarios, but it lacks the flexibility and composability offered by promises.
Promisification using the Promise Constructor
To promisify XHR requests, we can leverage the Promise constructor. This constructor takes a function with two arguments, resolve and reject, which can be thought of as callbacks for success and failure, respectively.
Let's update makeXHRRequest to use the Promise constructor:
function makeRequest(method, url) { return new Promise(function (resolve, reject) { var xhr = new XMLHttpRequest(); xhr.open(method, url); xhr.onload = function () { if (xhr.status >= 200 && xhr.status <p>This code initializes a new Promise, opens an XHR request, and handles both success and error scenarios.</p><p><strong>Chaining and Error Handling</strong></p><p>Promises provide a powerful way to chain multiple XHR requests and handle errors effectively. Here's an example of chaining requests and handling errors:</p><pre class="brush:php;toolbar:false">makeRequest('GET', 'https://www.example.com') .then(function (datums) { return makeRequest('GET', datums.url); }) .then(function (moreDatums) { console.log(moreDatums); }) .catch(function (err) { console.error('Augh, there was an error!', err.statusText); });
In this code, we're first making a GET request to 'example.com' and then, based on the response, making another GET request to a different endpoint (specified in the response). Any errors encountered during either request will be handled by the catch clause.
Custom Parameters and Headers
To make our XHR promisification more versatile, we can customize the parameters and headers. We'll introduce an opts object with the following signature:
{ method: String, url: String, params: String | Object, headers: Object, }
Here's a modified version of makeRequest that allows for custom parameters and headers:
function makeRequest(opts) { return new Promise(function (resolve, reject) { var xhr = new XMLHttpRequest(); xhr.open(opts.method, opts.url); xhr.onload = function () { if (xhr.status >= 200 && xhr.status <p>This version offers more flexibility in making XHR requests, allowing you to specify custom parameters and headers.</p><p>In conclusion, promisifying XHR requests using native promises is a straightforward approach that enhances the flexibility and composability of your frontend code. It empowers you to easily make asynchronous XHR requests, chain them, and handle errors effectively. By leveraging the concepts discussed in this article, you can unlock the potential of native promises for your frontend application development.</p>
The above is the detailed content of How Can I Simplify Native XHR Requests Using Promises?. For more information, please follow other related articles on the PHP Chinese website!
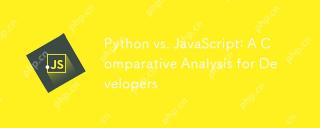
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
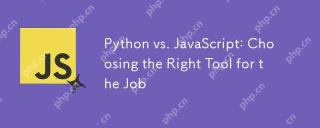
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
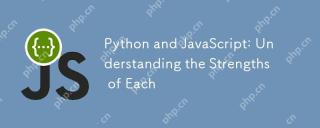
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
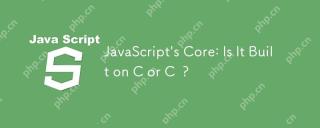
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
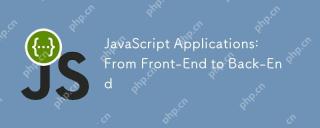
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
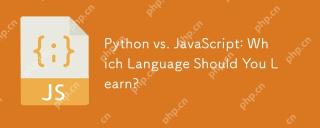
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
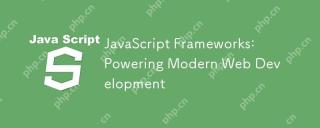
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
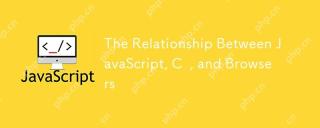
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
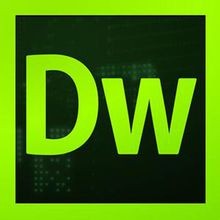
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
