Importing a .sql File into a MySQL Database Using PHP
When attempting to import a .sql file into a MySQL database using PHP, an error message is often encountered, reporting a problem during the import. This error message includes details about the database name, user name, password, host name, and import filename.
The code provided in the question utilizes the mysql_* functions, which have been deprecated as of PHP 5.5.0 and removed in PHP 7.0.0. Instead, alternative MySQL APIs such as mysqli or PDO_MySQL should be employed.
Alternative Import Method Using MySQL Client
Delegating the file import process to the MySQL client is generally a more reliable approach. MySQL provides command-line tools that can handle imports efficiently.
Improved PHP Code to Import .sql File
An alternative PHP code using a more appropriate import method is provided below:
<?php // File name $filename = 'churc.sql'; // MySQL host $mysql_host = 'localhost'; // MySQL username $mysql_username = 'root'; // MySQL password $mysql_password = ''; // Database name $mysql_database = 'dump'; // Establish MySQL connection $mysqli = new mysqli($mysql_host, $mysql_username, $mysql_password, $mysql_database); if ($mysqli->connect_error) { echo 'Error connecting to MySQL: ' . $mysqli->connect_error; exit; } // Read entire file $lines = file($filename); // Process each line foreach ($lines as $line) { // Skip comments and empty lines if (substr($line, 0, 2) == '--' || $line == '') { continue; } // Execute query if ($mysqli->query($line)) { echo 'Query successfully executed: ' . $line . '<br>'; } else { echo 'Error executing query: ' . $line . '<br>'; } } echo 'Tables imported successfully'; $mysqli->close(); ?>
This improved code utilizes the mysqli extension to connect to MySQL and execute the queries from the .sql file line by line.
The above is the detailed content of How Can I Safely Import a .sql File into MySQL Using PHP?. For more information, please follow other related articles on the PHP Chinese website!
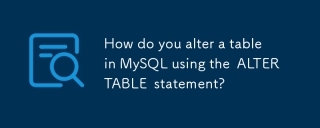
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
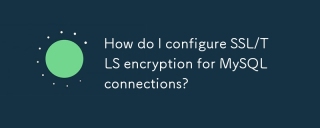
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
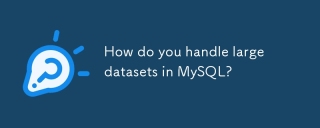
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
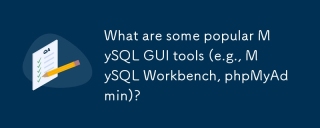
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
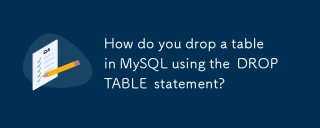
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
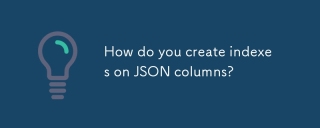
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
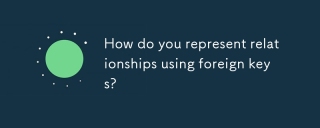
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
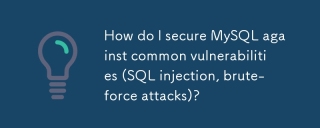
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
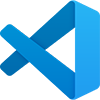
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
