How to Make an Image Move While Listening to a Keypress in Java
In Java, you can use a combination of Swing Timer and Key Bindings to make an image move while listening to a keypress. Here's how:
- Create a Swing Timer: A Swing Timer is used to periodically execute a task. In this case, you can use it to update the position of the image.
- Define Key Bindings: Key Bindings associate keys with specific actions. You can use key bindings to detect when a user presses a key and moves the image accordingly.
- Draw the Image: Override the paintComponent method of a JPanel to draw the image at its current position.
Example Code:
Here's an example code that demonstrates how to use a Swing Timer and Key Bindings to make an image move while listening to a keypress:
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class ImageMoveOnKeyPress extends JPanel implements ActionListener { private Image image; // The image to be moved private int x, y; // The current position of the image private Timer timer; // The Swing Timer to update the image position private KeyBindings keyBindings; // The Key Bindings to detect keypresses public ImageMoveOnKeyPress() { // Load the image image = Toolkit.getDefaultToolkit().getImage("image_path"); // Set the initial position of the image x = 50; y = 50; // Create a Swing Timer to update the image position timer = new Timer(10, this); // 10 milliseconds delay timer.start(); // Create Key Bindings to detect keypresses keyBindings = new KeyBindings(); getInputMap().put(KeyEvent.VK_LEFT, "left"); getInputMap().put(KeyEvent.VK_RIGHT, "right"); getInputMap().put(KeyEvent.VK_UP, "up"); getInputMap().put(KeyEvent.VK_DOWN, "down"); getActionMap().put("left", keyBindings.new MoveLeftAction()); getActionMap().put("right", keyBindings.new MoveRightAction()); getActionMap().put("up", keyBindings.new MoveUpAction()); getActionMap().put("down", keyBindings.new MoveDownAction()); } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); g.drawImage(image, x, y, null); } @Override public void actionPerformed(ActionEvent e) { // Update the image position based on keypress if (keyBindings.isLeftPressed()) { x -= 1; } else if (keyBindings.isRightPressed()) { x += 1; } else if (keyBindings.isUpPressed()) { y -= 1; } else if (keyBindings.isDownPressed()) { y += 1; } // Repaint the panel to display the moved image repaint(); } private class KeyBindings { private boolean leftPressed, rightPressed, upPressed, downPressed; public boolean isLeftPressed() { return leftPressed; } public void setLeftPressed(boolean leftPressed) { this.leftPressed = leftPressed; } public boolean isRightPressed() { return rightPressed; } public void setRightPressed(boolean rightPressed) { this.rightPressed = rightPressed; } public boolean isUpPressed() { return upPressed; } public void setUpPressed(boolean upPressed) { this.upPressed = upPressed; } public boolean isDownPressed() { return downPressed; } public void setDownPressed(boolean downPressed) { this.downPressed = downPressed; } private class MoveLeftAction extends AbstractAction { @Override public void actionPerformed(ActionEvent e) { leftPressed = true; } } private class MoveRightAction extends AbstractAction { @Override public void actionPerformed(ActionEvent e) { rightPressed = true; } } private class MoveUpAction extends AbstractAction { @Override public void actionPerformed(ActionEvent e) { upPressed = true; } } private class MoveDownAction extends AbstractAction { @Override public void actionPerformed(ActionEvent e) { downPressed = true; } } } public static void main(String[] args) { JFrame frame = new JFrame();
The above is the detailed content of How to Move an Image in Java Based on Key Presses?. For more information, please follow other related articles on the PHP Chinese website!
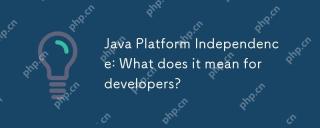
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
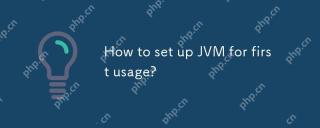
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
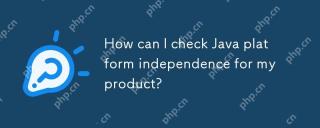
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss
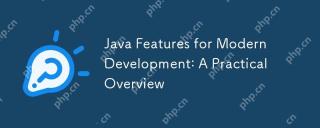
Javastandsoutinmoderndevelopmentduetoitsrobustfeatureslikelambdaexpressions,streams,andenhancedconcurrencysupport.1)Lambdaexpressionssimplifyfunctionalprogramming,makingcodemoreconciseandreadable.2)Streamsenableefficientdataprocessingwithoperationsli
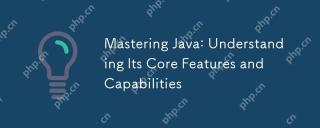
The core features of Java include platform independence, object-oriented design and a rich standard library. 1) Object-oriented design makes the code more flexible and maintainable through polymorphic features. 2) The garbage collection mechanism liberates the memory management burden of developers, but it needs to be optimized to avoid performance problems. 3) The standard library provides powerful tools from collections to networks, but data structures should be selected carefully to keep the code concise.
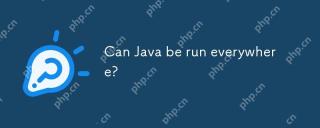
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
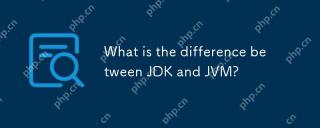
JDKincludestoolsfordevelopingandcompilingJavacode,whileJVMrunsthecompiledbytecode.1)JDKcontainsJRE,compiler,andutilities.2)JVMmanagesbytecodeexecutionandsupports"writeonce,runanywhere."3)UseJDKfordevelopmentandJREforrunningapplications.
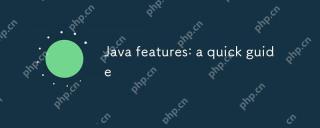
Key features of Java include: 1) object-oriented design, 2) platform independence, 3) garbage collection mechanism, 4) rich libraries and frameworks, 5) concurrency support, 6) exception handling, 7) continuous evolution. These features of Java make it a powerful tool for developing efficient and maintainable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
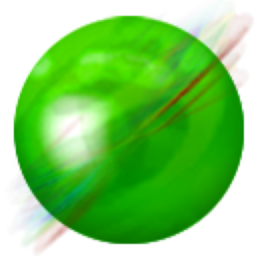
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
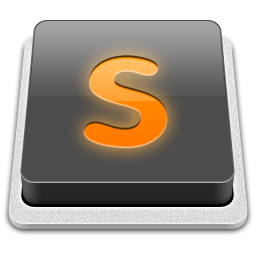
SublimeText3 Mac version
God-level code editing software (SublimeText3)
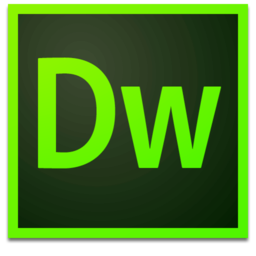
Dreamweaver Mac version
Visual web development tools
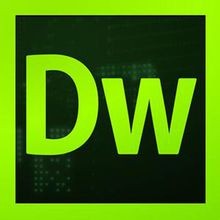
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
