


Can Java Handle SIGKILL Signals, and What's the Alternative for Graceful Shutdown?
SIGKILL Signal Handling in Java
The SIGKILL signal, often associated with the kill -9 command, cannot be handled or captured by any program in Java or any other language. This is an intentional design feature that ensures the ability to terminate even unresponsive or malicious processes.
Since SIGKILL is unmanageable, the focus should shift to handling the SIGTERM signal, which represents a controlled shutdown request. Java provides the Runtime.addShutdownHook() method to register shutdown hooks that will be executed when the program receives a SIGTERM or terminates gracefully.
Registering Shutdown Hooks
To register a shutdown hook:
Runtime.getRuntime().addShutdownHook(new Thread() { @Override public void run() { // Perform cleanup tasks System.out.println("Shutdown hook ran!"); } });
Example Program
The following test program demonstrates the use of shutdown hooks on OSX:
public class TestShutdownHook { public static void main(String[] args) throws InterruptedException { Runtime.getRuntime().addShutdownHook(new Thread() { @Override public void run() { System.out.println("Shutdown hook ran!"); } }); while (true) { Thread.sleep(1000); } } }
When you run this program with kill -15 (SIGTERM), it will print "Shutdown hook ran!" as expected.
Handling kill -9
As previously mentioned, kill -9 cannot be gracefully handled. However, you can implement a workaround using a wrapper script or a watcher program that monitors your main application. When the main application exits or is terminated, the watcher can perform cleanup tasks or notify other applications.
Example Wrapper Script
#!/usr/bin/env bash java TestShutdownHook wait # notify your other app that you quit echo "TestShutdownHook quit"
The above is the detailed content of Can Java Handle SIGKILL Signals, and What's the Alternative for Graceful Shutdown?. For more information, please follow other related articles on the PHP Chinese website!
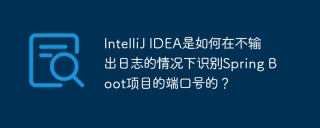
Start Spring using IntelliJIDEAUltimate version...
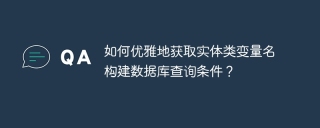
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
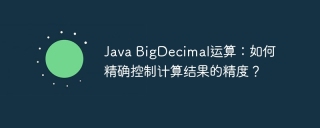
Java...
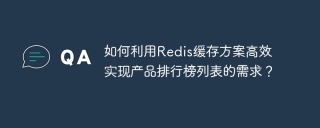
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
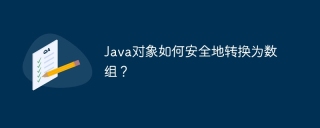
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
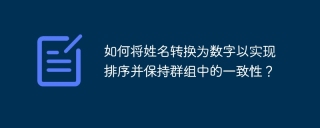
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
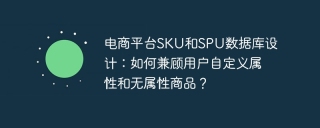
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
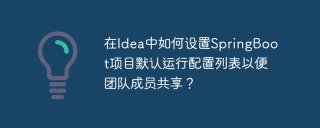
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.