


Tkinter - Geometry Management
Understanding Tkinter's Geometry Management
For effective GUI organization in Tkinter, it's important to understand the basic principles of its geometry management.
1. Top-level Windows
Start by configuring top-level windows with options such as:
- wm_geometry: Specify window size and screen position.
- wm_minsize and wm_maxsize: Set minimal and maximal window bounds.
- wm_resizable: Allow the user to resize the window.
- wm_attributes: Define attributes like topmost or fullscreen.
2. Arranging Child Widgets
Tkinter provides three geometry managers for arranging child widgets within a parent window:
a. Packer
Use the pack method to place widgets along the edges of the parent:
- fill: Expand the widget horizontally or vertically.
- expand: Fill the remaining parent space with additional widgets.
- side: Specify which edge the widget should be placed against.
- anchor: Position the widget within the allotted space.
b. Placer
Use the place method for fixed positioning:
- relheight and relwidth: Height and width relative to parent.
- relx and rely: Position relative to parent coordinates.
c. Gridder
Use the grid method for structured layout:
- columnspan and rowspan: Expand the widget to occupy multiple cells.
- sticky: Position the widget within its cell.
- grid_remove, grid_columnconfigure, and grid_rowconfigure: Advanced configuration options.
3. Choosing the Right Manager
Selecting the appropriate geometry manager depends on the complexity and requirements of your application:
- Packer: Quick and simple for aligning a few widgets.
- Placer: Suitable for single-page applications or background images.
- Gridder: Ideal for complex layouts with many widgets.
4. Optimizing Layouts
To enhance layout effectiveness, consider the following:
- Avoid Mixing Managers: Don't use grid and pack within the same master window.
- Nested Layouts: Create multiple frames and use different managers within each.
- Important Features: Refer to the documentation for specific manager options.
Example Code
The following code demonstrates a sample layout using different geometry managers:
import tkinter as tk # Root window root = tk.Tk() # Red frame holderframe = tk.Frame(root, bg='red') holderframe.pack() # Green display (Packer) display = tk.Frame(holderframe, width=600, height=25, bg='green') display.pack() # Orange display (Gridder) display2 = tk.Frame(holderframe, width=300, height=145, bg='orange') display2.grid(column=0, row=1) # Black display (Gridder) display3 = tk.Frame(holderframe, width=300, height=300, bg='black') display3.grid(column=1, row=1) # Yellow display (Gridder) display4 = tk.Frame(holderframe, width=300, height=20, bg='yellow') display4.grid(column=0, row=1) # Purple display (Placer) display5 = tk.Frame(holderframe, bg='purple') display5.place(x=0, y=170, relwidth=0.5, height=20) root.mainloop()
This code creates a layout with a red frame holding five child displays using different geometry managers, demonstrating the various ways to organize GUI elements in Tkinter.
The above is the detailed content of How do I effectively manage the geometry of widgets in Tkinter?. For more information, please follow other related articles on the PHP Chinese website!
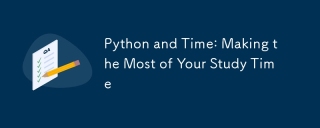
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
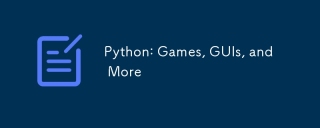
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
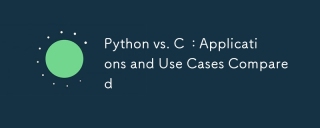
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
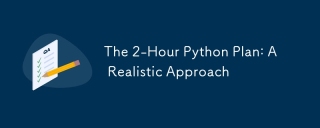
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
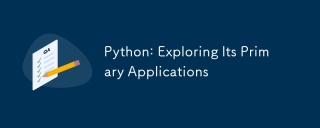
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
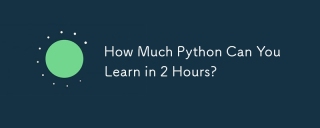
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
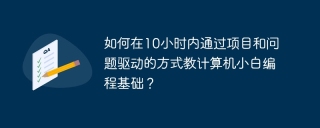
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
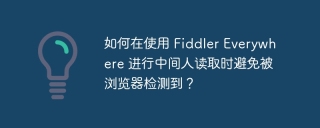
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
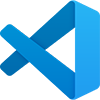
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
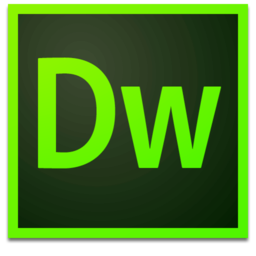
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor