


How to Retract a Salted Password from the Database and Authenticate a User
When implementing a membership site with salted passwords stored in a database, ensuring correct authentication becomes crucial. However, errors can arise when attempting to check for user existence.
The Issue
The code snippet below demonstrates an incorrect method of verifying if a member exists:
$name = mysqli_real_escape_string($connect, $_POST['name']); $password = mysqli_real_escape_string($connect, $_POST['password']); $saltQuery = "SELECT salt FROM users WHERE name = '$name';"; $result = mysqli_query($connect, $saltQuery); if ($result === false){ die(mysqli_error()); } $row = mysqli_fetch_assoc($result); $salt = $row['salt'];
This code attempts to retrieve the salt from the database but fails if the query returns false.
The Solution
To accurately verify a user's login credentials, we need to retrieve the stored password hash and compare it to the user's entered password. This process involves using the password_hash() and password_verify() functions.
Example Code for MySQLi
/** * mysqli example for a login with a stored password-hash */ $mysqli = new mysqli($dbHost, $dbUser, $dbPassword, $dbName); $mysqli->set_charset('utf8'); // Find the stored password hash in the database, searching by username $sql = 'SELECT password FROM users WHERE name = ?'; $stmt = $mysqli->prepare($sql); $stmt->bind_param('s', $_POST['name']); // it is safe to pass the user input unescaped $stmt->execute(); // If this user exists, fetch the password-hash and check it $isPasswordCorrect = false; $stmt->bind_result($hashFromDb); if ($stmt->fetch() === true) { // Check whether the entered password matches the stored hash. // The salt and the cost factor will be extracted from $hashFromDb. $isPasswordCorrect = password_verify($_POST['password'], $hashFromDb); }
Example Code for PDO
/** * pdo example for a login with a stored password-hash */ $dsn = "mysql:host=$dbHost;dbname=$dbName;charset=utf8"; $pdo = new PDO($dsn, $dbUser, $dbPassword); // Find the stored password hash in the database, searching by username $sql = 'SELECT password FROM users WHERE name = ?'; $stmt = $pdo->prepare($sql); $stmt->bindValue(1, $_POST['name'], PDO::PARAM_STR); // it is safe to pass the user input unescaped $stmt->execute(); // If this user exists, fetch the password hash and check it $isPasswordCorrect = false; if (($row = $stmt->fetch(PDO::FETCH_ASSOC)) !== false) { $hashFromDb = $row['password']; // Check whether the entered password matches the stored hash. // The salt and the cost factor will be extracted from $hashFromDb. $isPasswordCorrect = password_verify($_POST['password'], $hashFromDb); }
The above is the detailed content of How to Safely Authenticate Users with Salted Passwords Stored in a Database?. For more information, please follow other related articles on the PHP Chinese website!
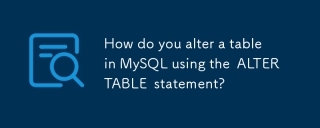
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
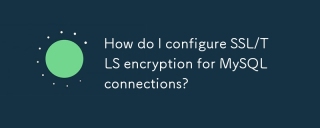
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
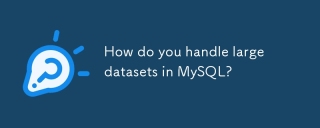
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
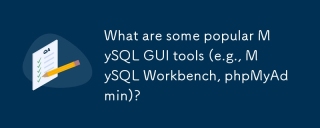
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
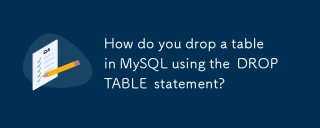
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
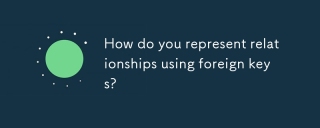
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
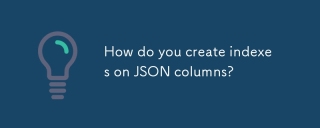
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
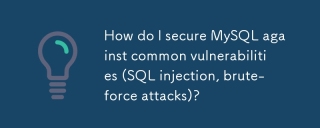
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
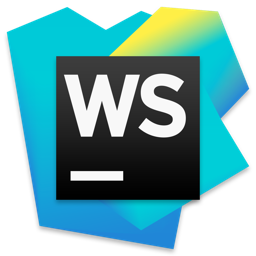
WebStorm Mac version
Useful JavaScript development tools
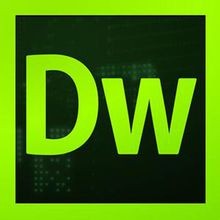
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
