Including Header Files versus Source Code
When working with multiple source files in a C program, it's crucial to understand the distinction between including header files (.h) and source code files (.cpp).
In the example provided, you encountered an error when including "foop.cpp" instead of "foop.h" in the main.cpp file. This is because header files are specifically designed to declare function prototypes and other necessary information, while source code files contain the actual function implementations.
Including Header Files
Including header files allows the compiler to locate and recognize functions defined in other source code files without duplicating the definitions. By including "foop.h," the main.cpp file gains access to the foo() function prototype, enabling the program to call it without knowing the full implementation details.
Including Source Code Files
In contrast, including "foop.cpp" directly would copy all the code from that file into the main.cpp file, leading to a multiple definition error. Since foo() is defined both in foop.cpp and main.cpp, the compiler becomes confused about which definition to use.
How It Works
When you include a header file, its contents are effectively copied into the current source code file. For instance, including "foop.h" would result in the following equivalent code in main.cpp:
// Header file contents int foo(int a); int main(int argc, char *argv[]) { // Rest of the main.cpp code }
However, including "foop.cpp" would lead to this:
// Source code file contents int foo(int a){ return ++a; } int main(int argc, char *argv[]) { // Rest of the main.cpp code }
Consequences
Including source code files directly can have unintended consequences, such as:
- Namespace pollution: Functions and variables defined in foop.cpp would become part of the main namespace, potentially conflicting with other global symbols.
- Increased compilation time: Including large source code files can slow down the compilation process.
- Error-prone: Manual inclusion of source code files is less reliable than using header files, which are automatically generated by the compiler.
Best Practice
Therefore, it's always good practice to include header files (.h) when referring to functions and declarations, and to keep source code implementation in separate files (.cpp). This ensures code modularity, avoids errors, and improves code readability.
The above is the detailed content of Why does including a .cpp file instead of a .h file in C lead to errors?. For more information, please follow other related articles on the PHP Chinese website!
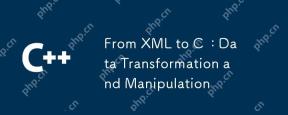
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
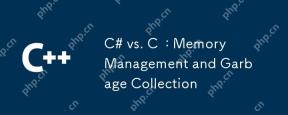
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
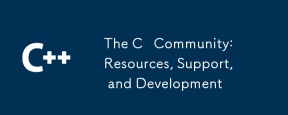
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
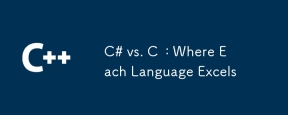
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
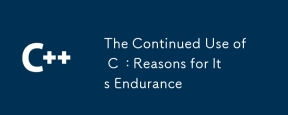
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
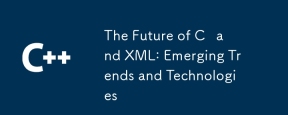
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
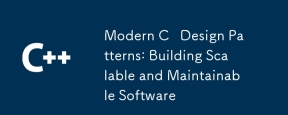
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
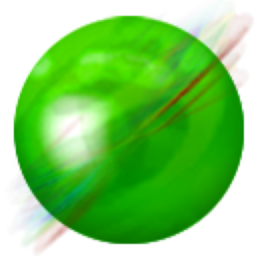
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor