Writing Efficient STL-Compliant Containers
Creating custom containers in accordance with the STL (Standard Template Library) guidelines ensures seamless integration with the STL ecosystem and guarantees predictable and consistent behavior. While the guidelines are not explicitly defined, adhering to the conventions of existing STL containers can guide your design.
The following template is a sample pseudo-container that demonstrates the core characteristics of an STL container:
template <class t class a="std::allocator<T">> class X { // Required Declarations: typedef A allocator_type; typedef typename A::value_type value_type; typedef typename A::reference reference; typedef typename A::iterator iterator; typedef typename A::const_iterator const_iterator; // Required Iterators: class iterator { // Required Iterator Declarations and Operators: iterator(); iterator(const iterator&); ~iterator(); iterator& operator=(const iterator&); // Comparison Operators: bool operator==(const iterator&) const; bool operator!=(const iterator&) const; bool operator() const; }; class const_iterator { // Required Const Iterator Declarations and Operators: const_iterator(); const_iterator(const const_iterator&); const_iterator(const iterator&); ~const_iterator(); const_iterator& operator=(const const_iterator&); // Comparison Operators: bool operator==(const const_iterator&) const; bool operator!=(const const_iterator&) const; bool operator() const; }; // Optional Reverse Iterators: typedef std::reverse_iterator<iterator> reverse_iterator; typedef std::reverse_iterator<const_iterator> const_reverse_iterator; // Required Member Functions: X(); X(const X&); ~X(); X& operator=(const X&); iterator begin(); const_iterator begin() const; const_iterator cbegin() const; iterator end(); const_iterator end() const; const_iterator cend() const; };</const_iterator></iterator></class>
To ensure the integrity of your containers, consider using a testing class like:
struct tester { friend verify; static int livecount; tester() { ++livecount; } tester(const tester&) { ++livecount; } ~tester() { assert(livecount); --livecount; } };
By testing your container with tester objects, you can verify that it follows the guidelines and behaves as expected.
The above is the detailed content of How to Efficiently Create STL-Compliant Custom Containers?. For more information, please follow other related articles on the PHP Chinese website!
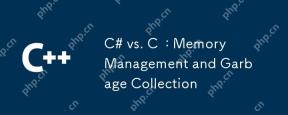
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
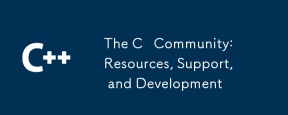
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
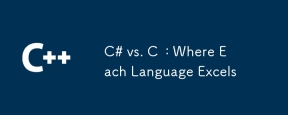
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
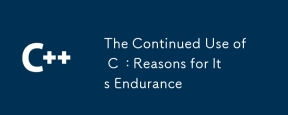
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
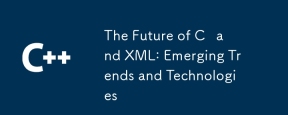
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
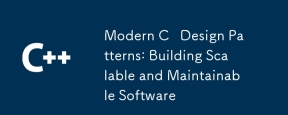
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
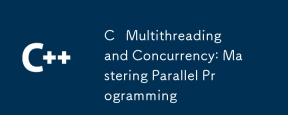
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
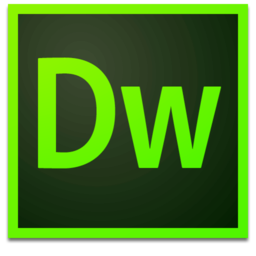
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.