


Managing Scheduled Functions with setInterval and clearInterval
Consider the following code fragment:
function doKeyDown(event) { switch (event.keyCode) { case 32: /* Space bar was pressed */ if (x == 4) { setInterval(drawAll, 20); } else { setInterval(drawAll, 20); x += dx; } break; } }
The goal is to call the drawAll function only once, not create a loop that repeatedly invokes it.
Using clearInterval to Stop Recurring Execution
setInterval establishes a recurring timer that calls the specified function periodically. To cease its execution, we can utilize clearInterval. Here's how it works:
var handle = setInterval(drawAll, 20); // When you want to stop it: clearInterval(handle); handle = 0; // Indication of an uncleared interval
On browsers, the handle is guaranteed to be a non-zero numerical value. Thus, setting handle = 0 serves as a convenient flag to indicate that the interval has been cleared.
Scheduling a One-time Function Call
If the intention is to execute a function only once, consider using setTimeout instead. This function schedules a function to be invoked after a specified time interval but doesn't continue firing it thereafter. It also returns a handle for potential cancellation.
setTimeout(drawAll, 20);
The above is the detailed content of How Can I Ensure a Function Called with `setInterval` Executes Only Once?. For more information, please follow other related articles on the PHP Chinese website!
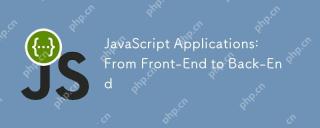
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
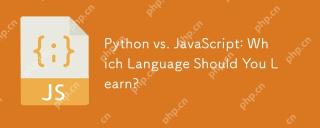
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
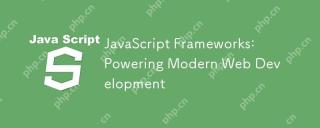
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
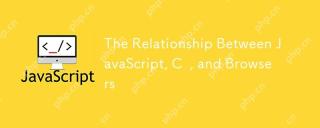
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
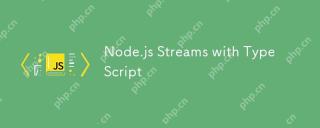
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
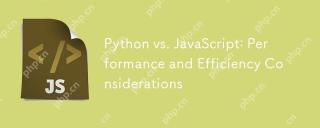
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
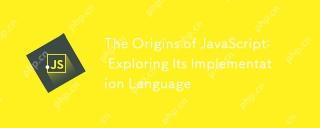
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
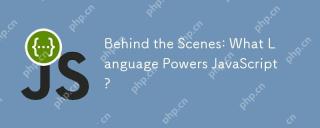
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
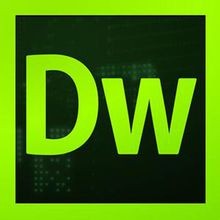
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
