MySQL Multiple Queries with PHP
In PHP, executing multiple MySQL queries in a single request can be challenging. This article addresses a common situation where multiple interdependent queries need to be combined for efficient processing.
The initial approach was to create individual views using the provided queries. However, this resulted in errors or empty result sets. To address this, PHP offers the mysqli_multi_query() function, which allows for the execution of concatenated queries.
Here's an example code snippet:
/* Create database connection */ $link = mysqli_connect("server", "user", "password", "database"); $query = "CREATE VIEW current_rankings AS SELECT * FROM main_table WHERE date = X;"; $query .= "CREATE VIEW previous_rankings AS SELECT rank FROM main_table WHERE date = date_sub('X', INTERVAL 1 MONTH);"; $query .= "CREATE VIEW final_output AS SELECT current_rankings.player, current_rankings.rank as current_rank LEFT JOIN previous_rankings.rank as prev_rank ON (current_rankings.player = previous_rankings.player);"; $query .= "SELECT *, @rank_change = prev_rank - current_rank as rank_change from final_output;"; /* Execute multiple queries using mysqli_multi_query() */ if (mysqli_multi_query($link, $query)) { do { /* Store current result set */ if ($result = mysqli_store_result($link)) { while ($row = mysqli_fetch_array($result)) { echo $row['player']. $row['current_rank']. $row['prev_rank']. $row['rank_change']; } mysqli_free_result($result); } } while (mysqli_next_result($link)); }
This approach combines all the queries into a single string and executes them concurrently. The mysqli_multi_query() function returns TRUE upon successful execution.
If the execution plan requires additional queries, they can be appended to the $query string with semicolons (;) as separators. Each result set can be stored in a separate variable using mysqli_store_result() and fetched using mysqli_fetch_array() within a loop.
Alternatively, you can also execute queries separately using the mysqli_query() function:
$query1 = "Create temporary table A select c1 from t1"; $result1 = mysqli_query($link, $query1) or die(mysqli_error()); $query2 = "select c1 from A"; $result2 = mysqli_query($link, $query2) or die(mysqli_error()); while($row = mysqli_fetch_array($result2)) { echo $row['c1']; }
This approach allows for greater control over query execution and result handling.
The above is the detailed content of How to Efficiently Execute Multiple MySQL Queries in PHP?. For more information, please follow other related articles on the PHP Chinese website!
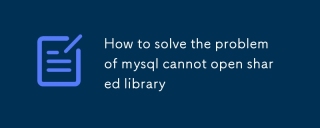
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
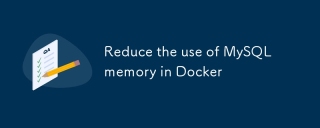
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
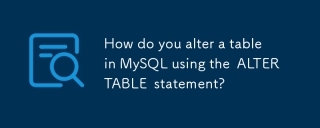
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
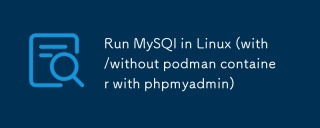
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
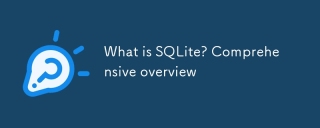
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
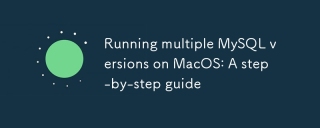
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
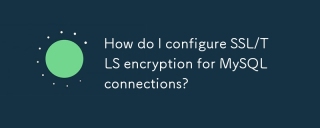
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
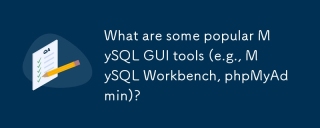
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
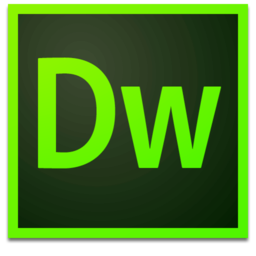
Dreamweaver Mac version
Visual web development tools
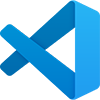
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
