Modules:
- Every python file is a module.Files which we save with the extension(.py) all are modules.
- Modules can be reused using import function-It helps to import one module to another module.
Special variables:
Denoted by "__"-Double underscore(in python it is called as dunder) in front and backside of a variable.
Example:1
Input:
print("Hello") print(__name__) print(__file__)
Output:
Hello __main__ /home/guru/Desktop/Guru/Bank.py
In the above example,
---> name is used to find whether we are working in same module or from different module.If we are working in same module then main will be the output which means in same working module we are printing.Incase if we are printing it in another module by importing then the output will be that module name.
--->file is used for locating the module.
Example:2
To prove module is reusable:
Case:1 Both the python modules are in same folder
Input:
calculator.py-module1
def add(no1,no2): print(no1+no2) def subtract(no1,no2): print(no1-no2) def multiply(no1,no2): print(no1*no2) def divide(no1,no2): print(no1/no2)
user.py-module2
import calculator calculator.add(10,3) calculator.multiply(10,3)
So we have imported from calculator.py to user.py and calling a function in module 2.
Output will be
13 30
Case:2 Python modules in different folders
If both modules are in different folders then the output will show modulenotfounderror.
Output:
ModuleNotFoundError: No module named 'calculator'
If we need specific functions alone from calculator.py means then no need to import whole module,instead we can use "from" to take specific function
from calculator import add, divide add(10,3) divide(10,2)
doc-->Documentation string
This variable is used to know about the particular module,like a description.
For every module ther will be a documentation which will be mentioned in ''' ''' or """ """.
'''It is about special variables''' print(__doc__)
Output:
It is about special variables
help-To see all details about the particular module like functions,file location, including documentation string.
#In user.py module: import calculator print(help(calculator))
Note:vi (module name.py) -is used to open the file in terminal itself instead of opening text editor.And after saving if we reload in text editor changes will be reflecting in it.
Type of modules:
userdefined-Whatever module we create with extension .py is userdefined modules.
predefined modules-Modules which are inbuilt in python.
help('modules') using this we can view all predefined modules in python.
Otp generator: Using random module:
import random otp = random.randint(100000,999999) print(otp)
Output:
263861 696781 802686
Task 1:
- Create a python module called Bank.
- Add functions: deposit(amount), withdraw(amount)
- Create one more python module called Customer
- From customer module, call deposit and withdraw functions of Bank module.
Bank.py:module 1
print("Hello") print(__name__) print(__file__)
customer.py:module 2
Hello __main__ /home/guru/Desktop/Guru/Bank.py
Output will be
def add(no1,no2): print(no1+no2) def subtract(no1,no2): print(no1-no2) def multiply(no1,no2): print(no1*no2) def divide(no1,no2): print(no1/no2)
Task:2
Few important predefined modules:
1) Os module:It is used for interacting with our operating system.
import calculator calculator.add(10,3) calculator.multiply(10,3)
output:
13 30
2) math: Performs mathematical operations.
Ex:Calculate square root
ModuleNotFoundError: No module named 'calculator'
Output:
from calculator import add, divide add(10,3) divide(10,2)
3) datetime: Manages dates and times.
'''It is about special variables''' print(__doc__)
Output:
It is about special variables
4) sys - System-Specific Parameters and Functions:Provides access to system-specific parameters.
#In user.py module: import calculator print(help(calculator))
output:Displays python version
import random otp = random.randint(100000,999999) print(otp)
5) re - Regular Expressions: Allows for pattern matching in strings.
If any string repeats and need to find that alone we can use re module.
263861 696781 802686
Output:
def deposit(amount): print("Total deposit amount is ",amount) return(amount) def withdraw(amount): print("Total withdrawal amount is ",amount) return(amount)
6) collections - Specialized Data Structures: Provides high-performance container datatypes.
import Bank total_deposit=Bank.deposit(100000) total_withdrawal=Bank.withdraw(20000) print("Bank balance is ",(total_deposit-total_withdrawal))
Output: From the above input output will count the occurances of each data and displays.
Total deposit amount is 100000 Total withdrawal amount is 20000 Bank balance is 80000
7) Django: Used to create web applications.
8) String: provides a collection of constants and functions that make it easier to work with strings.
Ex:#using one of the constants-string.ascii_lowercase
print("Contents:", os.listdir())
output:
Contents: ['user.py', 'Bank.py', '__pycache__', 'calculator.py', 'customer.py', 'hello.py', 'python classes']
The above is the detailed content of Python Day - odules-Meaning and Types,Tasks. For more information, please follow other related articles on the PHP Chinese website!
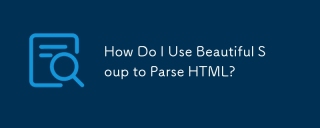
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
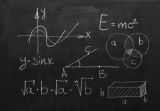
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
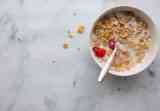
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
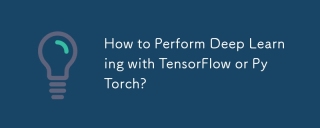
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
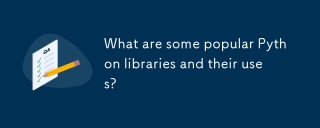
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
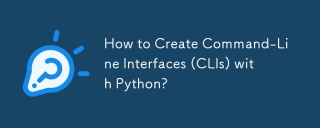
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
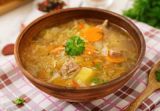
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
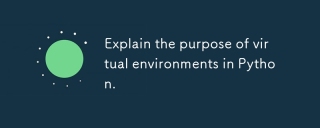
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
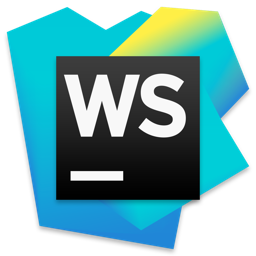
WebStorm Mac version
Useful JavaScript development tools
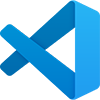
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
