Executing cv::warpPerspective for a Fake Deskewing on a Set of cv::Point
Question: How do I achieve a deskewing effect on a set of points using cv::warpPerspective? The points are not in a particular order and are stored within a vector.
Understanding the Issue:
- Incorrect point ordering: The order of points in the input and output vectors must match to achieve the desired transformation.
- Incorrect image size: The output image should have a width and height matching the bounding rectangle of the deskewed object.
Steps for Fake Deskewing:
- Correct Point Ordering: Ensure the order of points in both input and output vectors follows the same sequence (e.g., top-left, bottom-left, bottom-right, top-right).
- Rotated Rectangle Adjustment: Use cv::minAreaRect() to create a rotated rectangle around the input points. However, note that this method may slightly alter the original point coordinates.
- Affine Transform: Utilize the affine transform functions, cv::getAffineTransform() and cv::warpAffine(), as they are computationally more efficient for this specific deskewing operation.
- Different Output Size: To have the deskewed image contain only the object of interest, define a new image size (e.g., cv::Size(width, height)) that matches the bounding rectangle size.
- Apply Affine Transform: Pass the input image, input points, output points, and the defined output size to cv::warpAffine() to perform the actual deskewing transformation.
Example Code:
#include <opencv2> int main() { // Input image Mat src = imread("input.jpg"); // Input points (not in particular order) vector<point> points = { Point(408, 69), // Top-left Point(72, 2186), // Bottom-left Point(1584, 2426), // Bottom-right Point(1912, 291), // Top-right }; // Rotated rectangle (bounding box) RotatedRect boundingRect = minAreaRect(Mat(points)); // Corrected point ordering Point2f vertices[3]; vertices[0] = boundingRect.center + boundingRect.size * 0.5f; // Top-left vertices[1] = boundingRect.center + boundingRect.size * 0.5f; // Bottom-left vertices[1].y += boundingRect.size.height; vertices[2] = boundingRect.center - boundingRect.size * 0.5f; // Bottom-right // Output point ordering Point2f outputVertices[3]; outputVertices[0] = Point(0, 0); // Top-left outputVertices[1].x = outputVertices[0].x + boundingRect.size.width; // Bottom-left outputVertices[1].y = outputVertices[1].x; outputVertices[2] = outputVertices[0]; // Bottom-right // Affine transformation matrix Mat transformationMatrix = getAffineTransform(vertices, outputVertices); // Deskewed image with corrected size Mat deskewedImage; Size outputSize(boundingRect.size.width, boundingRect.size.height); warpAffine(src, deskewedImage, transformationMatrix, outputSize, INTER_LINEAR); // Save deskewed image imwrite("deskewed.jpg", deskewedImage); }</point></opencv2>
The above is the detailed content of How to Deskew a Set of Points Using cv::warpPerspective?. For more information, please follow other related articles on the PHP Chinese website!
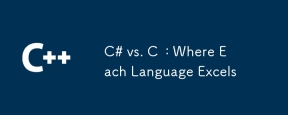
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
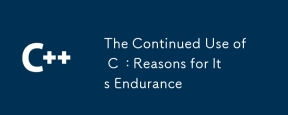
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
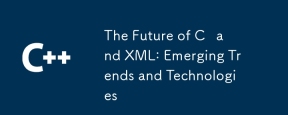
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
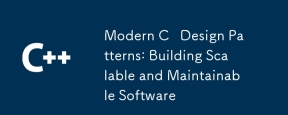
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
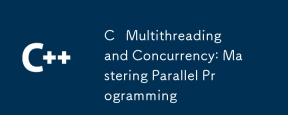
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
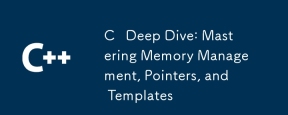
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
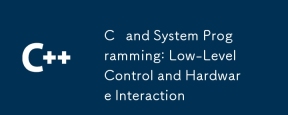
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
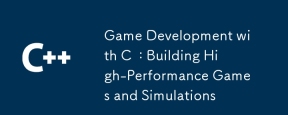
C is suitable for building high-performance gaming and simulation systems because it provides close to hardware control and efficient performance. 1) Memory management: Manual control reduces fragmentation and improves performance. 2) Compilation-time optimization: Inline functions and loop expansion improve running speed. 3) Low-level operations: Direct access to hardware, optimize graphics and physical computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.