


Execute JavaScript on User Typing Completion
Problem:
Detect when a user completes typing in a text box without triggering an action on every keystroke. This aims to minimize unnecessary AJAX requests while avoiding the requirement to press the enter button.
Solution:
To determine when a user has finished typing, a timer-based approach can be employed. Here's how it works with jQuery:
- Start a Timer on Key Release: When the user releases a key, trigger a timer to wait for a defined duration (e.g., 5 seconds).
- Clear the Timer on Key Press: If the user presses another key before the timer expires, clear the existing timer.
- Execute on Time Completion: When the timer expires without any further keypresses, it indicates the end of typing. Execute the intended action, such as an AJAX request.
Here's a code example:
// Setup variables var typingTimer; //timer identifier var doneTypingInterval = 5000; //time in ms, 5 seconds for example var $input = $('#myInput'); // Keyup: Start Timer $input.on('keyup', function () { clearTimeout(typingTimer); typingTimer = setTimeout(doneTyping, doneTypingInterval); }); // Keydown: Clear Timer $input.on('keydown', function () { clearTimeout(typingTimer); }); // Typing Completion: Execute Action function doneTyping () { // Perform the intended action, e.g., AJAX request }
By implementing this approach, you can optimize the performance of AJAX requests by reducing their frequency without the need for additional user input.
The above is the detailed content of How Can I Execute JavaScript Only After a User Finishes Typing in a Text Box?. For more information, please follow other related articles on the PHP Chinese website!
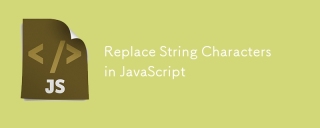
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
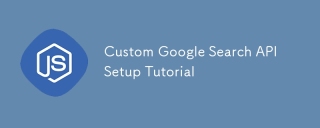
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
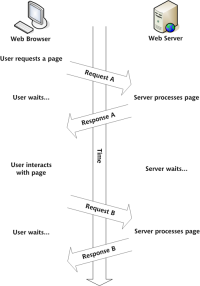
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
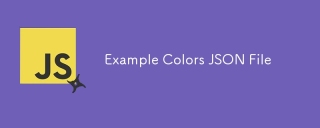
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
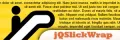
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
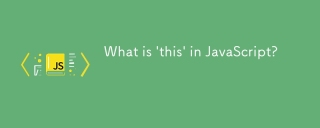
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
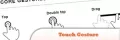
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
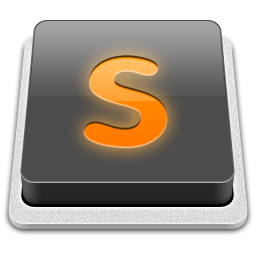
SublimeText3 Mac version
God-level code editing software (SublimeText3)
