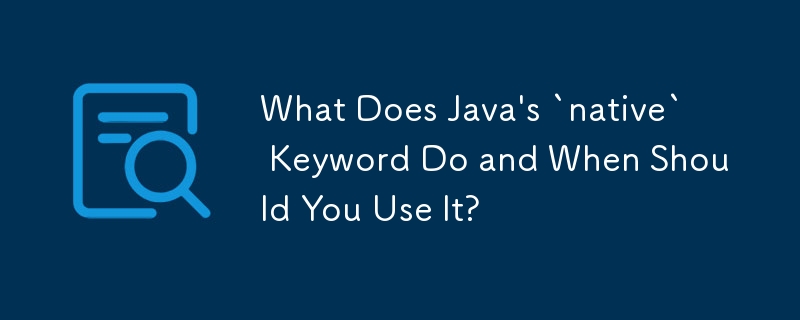
What's the Native Keyword in Java For?
The native keyword in Java allows you to call compiled, dynamically loaded libraries written in languages like C or C with arbitrary assembly code from within Java. This enables you to access lower-level functionality that may not be available in pure Java.
How it Works
To use native code in Java, you need to do the following:
- Create a header file (.h) that contains the function prototypes for the native methods you want to call.
- Implement the native methods in a source file (.c or .cpp).
- Compile the native code into a shared library(.so on Linux, .dll on Windows).
- Load the shared library into your Java program using System.loadLibrary().
- Use the native methods like any other Java method.
Example
Below is a simple example of a Java class that calls a native method written in C:
public class Example {
public native int square(int i);
public static void main(String[] args) {
System.loadLibrary("Example");
System.out.println(new Example().square(2));
}
}
Corresponding C code:
#include <jni.h>
JNIEXPORT jint JNICALL Java_Example_square(
JNIEnv *env, jobject obj, jint i) {
return i * i;
}
Benefits of Using Native Code
Using native code can provide several benefits, including:
-
Increased performance: Native code is often faster than Java code, as it can directly access hardware resources.
-
Access to low-level system functionality: Native code can perform tasks that are not possible in pure Java, such as interfacing with the operating system or accessing hardware devices.
-
Portability: Native code can be compiled for multiple platforms, making it easier to deploy your application across different systems.
Considerations
While using native code can be beneficial, it also comes with some considerations:
-
Increased complexity: Developing and maintaining native code can be more complex than using pure Java.
-
Reduced portability: Native code is compiled for a specific platform, which limits its portability.
-
Security risks: Writing native code requires a deep understanding of the underlying platform, and improper coding practices can introduce security risks.
The above is the detailed content of What Does Java\'s `native` Keyword Do and When Should You Use It?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn