Deterministic Generation of Unique Integers from Inputs
In seeking a solution for generating deterministic integers that avoid duplicates, the question revolves around finding a transformation method that maps input numbers to distinct outputs within a given range, such as an int64.
The answer lies in applying modular arithmetic derived from the Affine cipher:
f(P) = (mP + s) mod n
where:
- n = range of possible integers (e.g., 2^64 for int64)
- s
- m is coprime with n (meaning they share no common factors other than 1)
By choosing an appropriate m and s, this formula guarantees a unique mapping of input numbers to output integers within the specified range. For instance, for int64:
m = 39293 (any non-even number) s = 75321908 (any random number below 2^64)
With these values, the transform function:
func transform(p uint64) uint64 { return p*m + s }
ensures that each input integer produces a unique output integer, as demonstrated in the following Go playground example:
https://go.dev/play/p/EKB6SH3-SGu
For negative numbers, the logic remains the same. We can simply convert inputs and outputs between uint64 and int64 to maintain the unique mapping:
func signedTransform(p int64) int64 { return int64(transform(uint64(p))) }
This approach ensures that all possible integers within a given range are mapped to distinct output integers deterministically and without any collisions.
The above is the detailed content of How to Deterministically Generate Unique Integers from Inputs?. For more information, please follow other related articles on the PHP Chinese website!
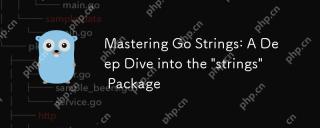
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
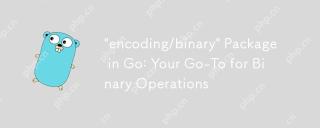
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
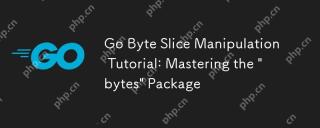
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
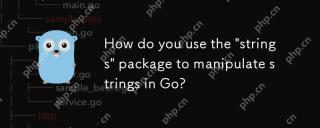
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
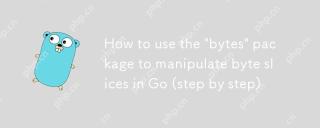
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
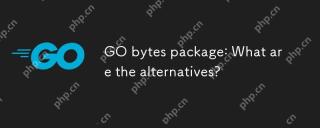
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
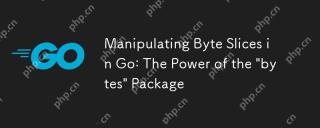
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
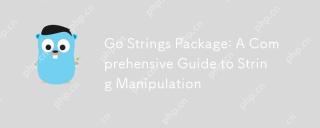
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
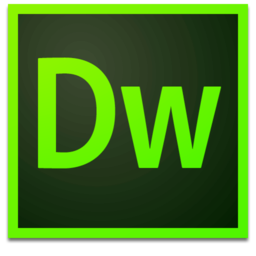
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
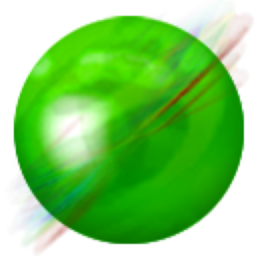
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
