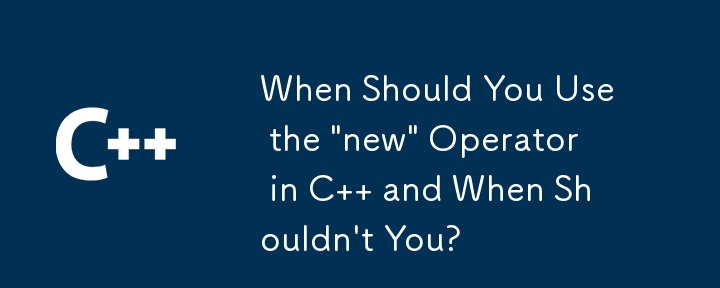
When to Utilize "new" and When Not to in C
In C , the usage of the "new" operator plays a crucial role in memory management and object instantiation. Understanding when to employ it is essential for effective coding.
When to Use "new"
-
Object Persistence: When you require an object to exist beyond its scope, employ "new." The "delete" operator will dispose of the object after use. This is necessary to prevent automatic object destruction when the scope ends.
-
Array Allocation: Dynamically sized arrays cannot be allocated in-place or on the stack. "new" enables the allocation of variable-sized arrays at runtime.
When Not to Use "new"
-
Simple Object Instantiation: For basic objects that should be discarded after their scope ends, direct instantiation without "new" is sufficient. The object will be automatically destroyed upon exiting its scope.
-
In-Place Allocation within Classes: Member variables within classes are implicitly allocated on the heap or stack based on the containing object's location. "new" is not needed for in-place allocation within classes.
Example:
Consider the following code:
Point p1 = Point(0, 0); // Instantiation without "new"
Point* p2 = new Point(0, 0); // Instantiation with "new"
- p1 will undergo automatic destruction when its scope ends.
- p2 will remain allocated on the heap until the delete operator is used.
Conclusion:
Understanding when to use and not use "new" in C is essential for efficient memory management and object lifecycle control. By following the guidelines discussed, you can effectively handle dynamic object creation and avoid potential memory leaks or scope-related issues.
The above is the detailed content of When Should You Use the 'new' Operator in C and When Shouldn't You?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn