


How Can I Ensure All Goroutines Finish When Using a Buffered Channel as a Semaphore?
Waiting for Buffered Channel Emptiness Using a WaitGroup
In concurrent programming, it's often necessary to control the number of concurrently executing goroutines. A common approach is to use a buffered channel as a semaphore. However, it's important to note the limitations of this approach.
Consider the following example:
ints := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10} sem := make(chan struct{}, 2) for _, i := range ints { sem<p>This code uses a buffered channel (sem) of size 2 to limit the number of concurrent goroutines to 2. However, there's a problem: the program may end before the last few goroutines have completed their tasks. This is because the buffered channel can become empty at any point during processing, even while the program is dispatching more goroutines.</p><p>To wait for a buffered channel to drain, we cannot rely on the channel itself. Instead, we can use a sync.WaitGroup to track the number of outstanding goroutines:</p><pre class="brush:php;toolbar:false">sem := make(chan struct{}, 2) var wg sync.WaitGroup for _, i := range ints { wg.Add(1) sem<p>In this modified code, we use wg.Add(1) to increment the wait group before dispatching each goroutine. The defer wg.Done() statement then decrements the wait group when the goroutine completes its task. Finally, wg.Wait() blocks until the wait group reaches zero, ensuring that all goroutines have finished executing. This approach allows us to wait for the channel to drain and ensures that the program doesn't terminate prematurely.</p>
The above is the detailed content of How Can I Ensure All Goroutines Finish When Using a Buffered Channel as a Semaphore?. For more information, please follow other related articles on the PHP Chinese website!
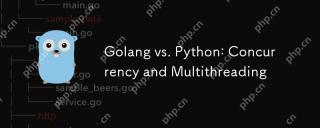
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
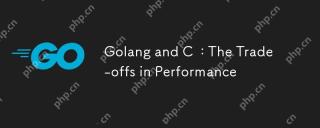
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
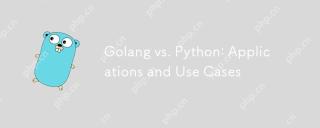
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
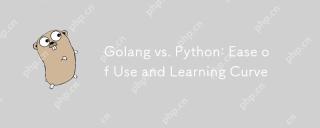
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
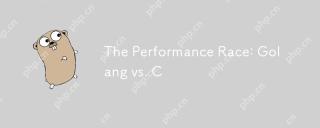
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
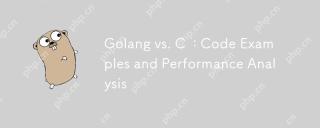
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
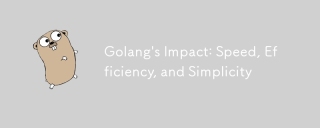
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
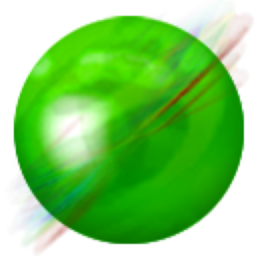
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
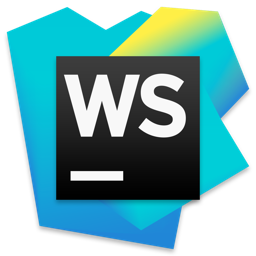
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version